Python 中如何判断两个列表有相同交集
时间: 2023-08-10 08:05:35 浏览: 77
可以使用 Python 内置的 set() 数据类型,将列表转换为集合,然后使用交集操作符 & 来判断两个集合是否有相同的元素。示例如下:
```python
list1 = [1, 2, 3, 4, 5]
list2 = [4, 5, 6, 7, 8]
set1 = set(list1)
set2 = set(list2)
if set1 & set2:
print("两个列表有相同交集")
else:
print("两个列表没有相同交集")
```
输出结果为:
```
两个列表有相同交集
```
其中,set() 函数可以将列表转换为集合,& 操作符可以求两个集合的交集。
相关问题
python 判断两个列表有多少相同元素
在 Python 中,判断两个列表中有多少相同元素可以使用集合(set)数据结构来完成。集合是一种无序且不包含重复元素的数据结构。你可以将两个列表转化为集合,然后求它们的交集,最后得到的就是相同的元素个数。以下是步骤:
```python
def count_common_elements(list1, list2):
set1 = set(list1)
set2 = set(list2)
common_elements = set1 & set2 # 使用 "&" 运算符获取交集
return len(common_elements)
list1 = [1, 2, 3, 4, 5]
list2 = [4, 5, 6, 7, 8]
print(count_common_elements(list1, list2))
```
如果你需要返回相同元素的具体列表,可以稍微修改一下函数:
```python
def find_common_elements(list1, list2):
set1 = set(list1)
set2 = set(list2)
common_elements = set1 & set2
return list(common_elements)
# 示例
common_list = find_common_elements(list1, list2)
print("Common elements:", common_list)
```
python找出两个列表中的相同元素
在Python中,你可以通过多种方法找到两个列表中的相同元素。以下是几种常见的方法:
1. **列表推导式**:
```python
list1 = [1, 2, 3, 4, 5]
list2 = [4, 5, 6, 7, 8]
common_elements = [element for element in list1 if element in list2]
```
2. **set交集**:
```python
list1 = [1, 2, 3, 4, 5]
list2 = [4, 5, 6, 7, 8]
common_elements = list(set(list1) & set(list2))
```
这里先将列表转换为集合,然后计算它们的交集,再转回列表。
3. **循环遍历**:
```python
def find_common(lst1, lst2):
common = []
for item in lst1:
if item in lst2:
common.append(item)
return common
list1 = [1, 2, 3, 4, 5]
list2 = [4, 5, 6, 7, 8]
result = find_common(list1, list2)
```
这种方法逐个检查每个元素是否存在于另一个列表中。
以上每种方法都会返回一个包含两个列表中共有元素的新列表。如果想要直接打印出来,可以直接查看`common_elements`或`result`变量的内容。
阅读全文
相关推荐
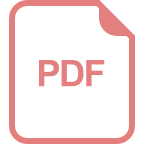
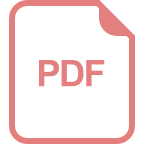
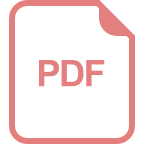













