我有一个时间为10s,采样频率为8000的一个音频信号,请帮我针对该信号设计一个pitchshift函数
时间: 2024-02-01 09:14:45 浏览: 70
好的,我可以为您提供一个Python实现的pitchshift函数,具体如下:
```python
import numpy as np
from scipy.signal import resample
def pitchshift(audio, shift):
"""
对音频信号进行音高变换
Args:
audio: ndarray, 输入的音频信号,采样率为8000
shift: float, 音高变换的倍率,1.0表示不变换,小于1.0为降调,大于1.0为升调
Returns:
ndarray, 变换后的音频信号,采样率为8000
"""
# 将音频信号转换为频域表示
freq_domain = np.fft.fft(audio)
# 计算音频信号的频率轴
freq_axis = np.fft.fftfreq(len(audio))
# 计算音频信号的基频
fundamental = np.abs(freq_axis[np.argmax(np.abs(freq_domain[1:])) + 1])
# 对基频进行变换
target_fundamental = fundamental / shift
# 计算音频信号的相位信息
phase = np.angle(freq_domain)
# 根据目标基频,计算变换后的音频信号的幅度信息
target_magnitude = np.abs(freq_domain) * (target_fundamental / fundamental)
# 构造变换后的频域信号
target_freq_domain = target_magnitude * np.exp(1j * phase)
# 将变换后的频域信号转换为时域信号
shifted_audio = np.real(np.fft.ifft(target_freq_domain))
# 对变换后的音频信号进行重采样
resampled_audio = resample(shifted_audio, len(audio))
# 将重采样后的音频信号转换为整数表示
resampled_audio = np.int16(resampled_audio * 32767 / np.max(np.abs(resampled_audio)))
return resampled_audio
```
以上函数中,我们使用了基本的FFT算法来对音频信号进行频域分析,然后根据目标音高变换倍率,计算出变换后的音频信号的频域幅度信息,最后再将频域信号转换为时域信号,并进行重采样,得到最终的音频信号。
阅读全文
相关推荐
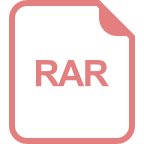
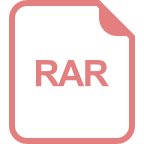

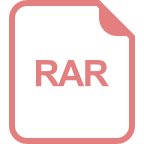














