用python随机生成100个2-1000范围内的整数,将生成的原始数据存入yuanshi列表,将素数存入sushu列表,将和数存入heshu列表,如有重复的数据,存入chongfu列表,并进行打印;
时间: 2023-06-14 10:05:03 浏览: 67
可以使用Python中的random和math库来生成随机整数和判断素数。代码如下:
```python
import random
import math
yuanshi = []
sushu = []
heshu = []
chongfu = []
for i in range(100):
num = random.randint(2, 1000)
yuanshi.append(num)
if num in heshu:
chongfu.append(num)
else:
heshu.append(num)
if num < 2:
continue
is_prime = True
for j in range(2, int(math.sqrt(num))+1):
if num % j == 0:
is_prime = False
break
if is_prime:
sushu.append(num)
print("原始数据:", yuanshi)
print("素数:", sushu)
print("和数:", heshu)
print("重复数据:", chongfu)
```
注:代码中的判断素数算法采用了较为简单的试除法,可能会对比较大的数进行多次循环判断,不够高效,但在本题数据范围内效果还是可以的。
相关问题
Traceback (most recent call last): File "D:\360MoveData\Users\Norah\Desktop\yuanshi3\xingtuchuli.py", line 158, in <module> img = Image.fromarray(img_translated_rotated) #src是numpy数组,转化为PIL能处理的图像 File "C:\Users\Norah\AppData\Local\Programs\Python\Python39\lib\site-packages\PIL\Image.py", line 3112, in fromarray return frombuffer(mode, size, obj, "raw", rawmode, 0, 1) File "C:\Users\Norah\AppData\Local\Programs\Python\Python39\lib\site-packages\PIL\Image.py", line 3028, in frombuffer return frombytes(mode, size, data, decoder_name, args) File "C:\Users\Norah\AppData\Local\Programs\Python\Python39\lib\site-packages\PIL\Image.py", line 2970, in frombytes im.frombytes(data, decoder_name, args) File "C:\Users\Norah\AppData\Local\Programs\Python\Python39\lib\site-packages\PIL\Image.py", line 822, in frombytes s = d.decode(data) TypeError: a bytes-like object is required, not 'Image'
这个错误提示是因为你调用了 PIL 库中的 fromarray 函数,但是传入的参数类型不正确。fromarray 函数需要传入一个 numpy 数组作为参数,但是你传入的是一个 Image 对象。建议你检查一下代码,看看是不是在调用 fromarray 函数之前,你对 img_translated_rotated 进行了一些不正确的操作,导致它的数据类型变化了。你可以尝试使用 numpy 的 astype 函数将 img_translated_rotated 转换为正确的数据类型。具体操作可以参考下面的代码示例:
```python
import numpy as np
from PIL import Image
# 假设你的原始图像是 img,你已经对它进行了一些操作,得到了 img_translated_rotated
img_translated_rotated = ...
# 使用 astype 函数将 img_translated_rotated 转换为正确的数据类型
img_translated_rotated = img_translated_rotated.astype(np.uint8)
# 将 numpy 数组转换为 PIL 图像
img = Image.fromarray(img_translated_rotated)
```
``` def z_score(yuanshi, arrange,sd): return(yuanshi-arrange)/(sd) result = z_score(200,100,9) print(result) ```
优化说明:
1. 函数名`z_score`应该使用英文命名,以提高代码的可读性。
2. 参数名`yuanshi`、`arrange`和`sd`应该使用英文命名,并且更加明确地表示它们的含义。例如,`yuanshi`可以改为`value`,`arrange`可以改为`mean`,`sd`可以改为`standard_deviation`。
3. 函数体内的除法操作应该添加括号,以避免运算优先级错误。
4. 为了代码的健壮性,可以在函数中添加参数校验,确保传入的参数是正确的。
优化后的代码:
```python
def calculate_z_score(value, mean, standard_deviation):
if standard_deviation <= 0:
raise ValueError("标准差必须大于0")
return (value - mean) / standard_deviation
result = calculate_z_score(200, 100, 9)
print(result)
```
优化后的代码更加清晰和健壮,函数参数使用了英文命名,并且对标准差进行了非负校验,以避免计算错误。
阅读全文
相关推荐
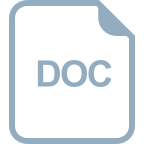
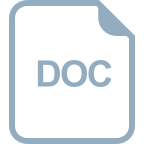
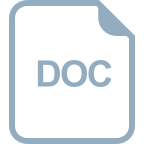
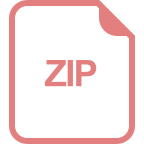
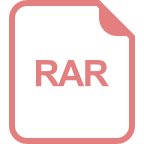
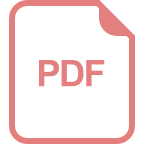
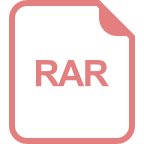
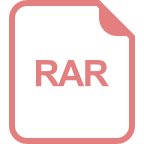
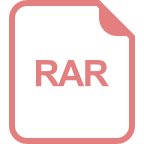





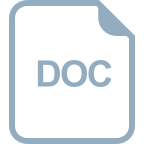
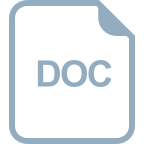
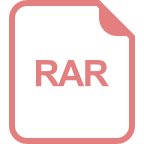