2.凯撒加密的编程逻辑 加密公式: f(a)=(a+N) mod 26 解密公式: f(a)=(a+(26-N)) mod 26 其中N代表的是位移数,也可以算是k; 3.凯撒加密的算法实现用完整C语言代码实现
时间: 2024-11-27 18:15:49 浏览: 0
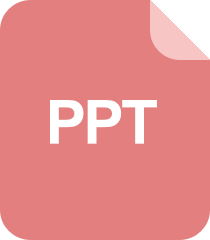
常见加密算法讲解.ppt

凯撒加密,也称作Caesar Cipher,是一种简单的替换式加密技术,通过将明文中的每个字母按照字母表顺序向后(如果是加密)或向前(如果是解密)移动固定数量的位置来进行编码。这种算法常用于教学中展示基本的密码学原理。
编程逻辑的实现可以分为两个步骤:加密和解密函数。
```c
#include <stdio.h>
#include <string.h>
// 加密函数
char caesar_encrypt(char c, int shift) {
if ('A' <= c && c <= 'Z') { // 处理大写字母
return ((c - 'A' + shift) % 26) + 'A';
} else if ('a' <= c && c <= 'z') { // 处理小写字母
return ((c - 'a' + shift) % 26) + 'a';
}
return c; // 非字母字符不变
}
// 解密函数
char caesar_decrypt(char c, int shift) {
return caesar_encrypt(c, -shift);
}
int main() {
char plaintext[100], ciphertext[100];
int shift;
printf("请输入明文:\n");
fgets(plaintext, sizeof(plaintext), stdin);
plaintext[strcspn(plaintext, "\n")] = '\0'; // 移除行尾换行符
printf("请输入位移数N(k):\n");
scanf("%d", &shift);
for (int i = 0; plaintext[i]; i++) {
ciphertext[i] = caesar_encrypt(plaintext[i], shift);
}
ciphertext[strcspn(ciphertext, "\n")] = '\0'; // 移除结束后的空字符
printf("加密后的密文为: %s\n", ciphertext);
printf("输入密文以解密:\n");
fgets(ciphertext, sizeof(ciphertext), stdin);
ciphertext[strcspn(ciphertext, "\n")] = '\0';
for (int i = 0; ciphertext[i]; i++) {
plaintext[i] = caesar_decrypt(ciphertext[i], shift);
}
plaintext[strcspn(plaintext, "\n")] = '\0';
printf("解密后的原文为: %s\n", plaintext);
return 0;
}
```
阅读全文
相关推荐
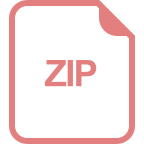
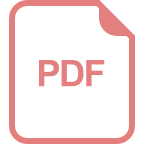

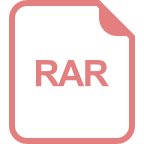
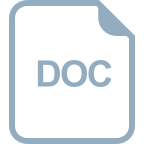
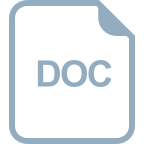
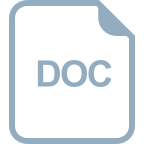
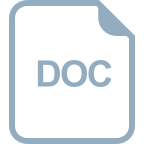
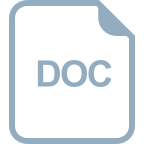
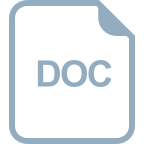
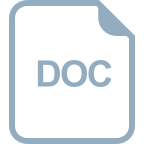
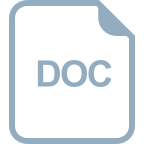



