用C语言完整编程实现以下功能:1、通过键盘输入表中元素值,利用尾插法建单链表,并返回该单链表头指针L :2、将链表L中的元素就地逆置。
时间: 2024-12-06 16:21:40 浏览: 23
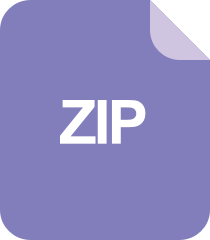
尾插法建立不带头结点的单链表(C语言).zip
在C语言中,我们可以使用结构体来表示链表节点,并通过函数分别实现插入和逆置操作。这里是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新节点并插入到链表尾部
Node* insert(int value, Node** L) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = value;
newNode->next = *L; // 如果链表为空,则新节点直接指向它
*L = newNode; // 更新链表头指针
return newNode;
}
// 将链表逆置
void reverse(Node** L) {
Node* prev = NULL;
Node* current = *L;
Node* nextTemp;
while (current != NULL) {
nextTemp = current->next;
current->next = prev;
prev = current;
current = nextTemp;
}
*L = prev; // 逆置后的新头指针
}
int main() {
Node* L = NULL;
// 输入并插入元素
int num;
while (scanf("%d", &num) == 1 && num != -1) {
L = insert(num, &L);
}
// 输出原始链表(仅用于测试)
printf("Original list:\n");
Node* temp = L;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
// 逆置链表
reverse(&L);
// 输出逆置后的链表
printf("Reversed list:\n");
temp = L;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
return 0;
}
```
在这个程序中,`insert` 函数负责接收用户输入并将数据添加到链表的尾部,而 `reverse` 函数则遍历链表,每次改变当前节点的 `next` 指针使其指向前一个节点,最终完成链表的逆置。
阅读全文
相关推荐
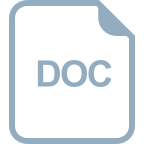
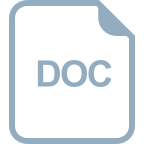












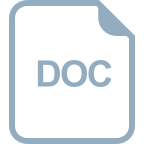
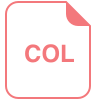


