由尾插法构建单链表c语言
时间: 2024-10-19 15:07:46 浏览: 13
在C语言中,可以使用尾插法来构建单链表。尾插法是指在链表的末尾添加新的节点,以维护链表的顺序。下面是一个使用尾插法构建单链表的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct Node {
int data;
struct Node* next;
};
// 创建新节点
struct Node* createNode(int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 尾插法构建单链表
struct Node* createLinkedList(int arr[], int n) {
struct Node* head = NULL; // 头节点指针
for (int i = n - 1; i >= 0; i--) { // 从数组末尾开始遍历
struct Node* newNode = createNode(arr[i]); // 创建新节点
if (head == NULL) { // 如果链表为空,将新节点作为头节点
head = newNode;
} else { // 否则将新节点插入到链表末尾
struct Node* cur = head; // 当前节点指针
while (cur->next != NULL) { // 找到链表末尾节点
cur = cur->next;
}
cur->next = newNode; // 将新节点插入到链表末尾
}
}
return head; // 返回链表头节点指针
}
// 打印链表
void printList(struct Node* head) {
struct Node* cur = head;
while (cur != NULL) {
printf("%d ", cur->data);
cur = cur->next;
}
printf("\n");
}
int main() {
int arr[] = {1, 2, 3, 4, 5}; // 要添加到链表的数组元素
int n = sizeof(arr) / sizeof(int); // 数组元素个数
struct Node* head = createLinkedList(arr, n); // 构建链表并返回头节点指针
printList(head); // 打印链表内容
return 0;
}
```
在上面的代码中,我们首先定义了一个链表节点结构体`Node`,包含一个数据域`data`和一个指向下一个节点的指针`next`。然后,我们实现了两个函数`createNode`和`createLinkedList`,分别用于创建新节点和构建单链表。在`createLinkedList`函数中,我们从数组的末尾开始遍历,依次创建新节点并将其插入到链表的末尾。最后,我们实现了`printList`函数用于打印链表的内容。在主函数中,我们使用数组元素构建了一个单链表,并打印了其内容。
阅读全文
相关推荐
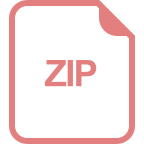
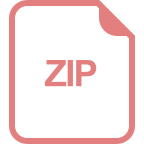
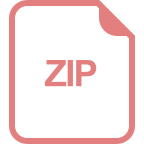










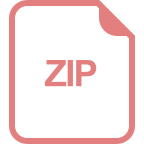
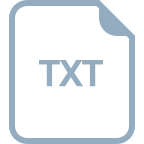
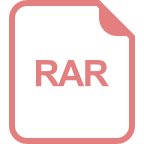
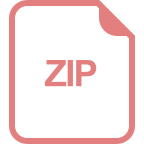
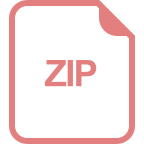
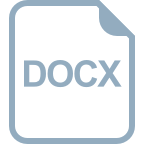