transformer模型预测代码
时间: 2023-07-27 13:06:55 浏览: 113
Sure, here is an example code snippet for using the Transformer model to make predictions:
```python
import torch
from torch import nn
from torch.nn import Transformer
from torch.nn import TransformerEncoder, TransformerEncoderLayer
# Define the Transformer model
class TransformerModel(nn.Module):
def __init__(self, ntoken, ninp, nhead, nhid, nlayers):
super(TransformerModel, self).__init__()
self.model_type = 'Transformer'
self.src_mask = None
self.encoder = nn.Embedding(ntoken, ninp)
self.pos_encoder = PositionalEncoding(ninp)
encoder_layers = TransformerEncoderLayer(ninp, nhead, nhid)
self.transformer_encoder = TransformerEncoder(encoder_layers, nlayers)
self.fc = nn.Linear(ninp, ntoken)
self.init_weights()
def init_weights(self):
initrange = 0.1
self.encoder.weight.data.uniform_(-initrange, initrange)
self.fc.bias.data.zero_()
self.fc.weight.data.uniform_(-initrange, initrange)
def forward(self, src):
src = self.encoder(src) * math.sqrt(self.ninp)
src = self.pos_encoder(src)
output = self.transformer_encoder(src)
output = self.fc(output)
return output
# Initialize the Transformer model
ntoken = 1000 # number of tokens in your vocabulary
ninp = 256 # embedding dimension
nhead = 8 # number of attention heads
nhid = 512 # hidden layer dimension
nlayers = 6 # number of Transformer layers
model = TransformerModel(ntoken, ninp, nhead, nhid, nlayers)
# Load pre-trained weights if available
model.load_state_dict(torch.load('transformer_model.pt'))
# Set the model to evaluation mode
model.eval()
# Prepare input data
input_data = torch.tensor([[1, 2, 3, 4, 5]]) # replace with your input sequence
# Make predictions
with torch.no_grad():
output = model(input_data)
# Get the predicted token indices
predicted_indices = torch.argmax(output, dim=2)
# Print the predicted token indices
print(predicted_indices)
```
Please note that this code is a basic example and may need modifications based on your specific use case. Additionally, make sure to replace the placeholders with your own data and adjust the hyperparameters according to your needs.
阅读全文
相关推荐
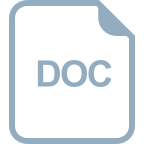
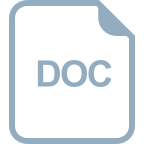
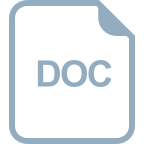















