使用estimators应该导入什么
时间: 2024-01-24 21:17:00 浏览: 27
在使用 TensorFlow Estimator API 时,你需要导入以下模块:
```python
import tensorflow as tf
from tensorflow import estimator
```
其中, `tf` 是 TensorFlow 库, `estimator` 是 TensorFlow Estimator API 的核心模块。
相关问题
n_classes = 3 n_estimators = 30 plot_colors = "ryb" cmap = plt.cm.RdYlBu plot_step = 0.02 # fine step width for decision surface contours plot_step_coarser = 0.5 # step widths for coarse classifier guesses RANDOM_SEED = 13 # fix the seed on each iteration iris = load_iris() plot_idx = 1 models = [DecisionTreeClassifier(max_depth=None), RandomForestClassifier(n_estimators=n_estimators), ExtraTreesClassifier(n_estimators=n_estimators), AdaBoostClassifier(DecisionTreeClassifier(max_depth=3), 将上面的代码加上注释
下面是对该代码的注释:
```python
# 导入需要的库和模块
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
from sklearn.ensemble import RandomForestClassifier, ExtraTreesClassifier, AdaBoostClassifier
import matplotlib.pyplot as plt
import numpy as np
# 定义需要的参数
n_classes = 3 # 类别数
n_estimators = 30 # 集成模型中基分类器的数量
plot_colors = "ryb" # 绘图时使用的颜色
cmap = plt.cm.RdYlBu # 绘图时使用的颜色映射
plot_step = 0.02 # 决策面轮廓线的细度
plot_step_coarser = 0.5 # 粗略分类器猜测的步骤宽度
RANDOM_SEED = 13 # 每次迭代时固定种子
# 加载鸢尾花数据集
iris = load_iris()
# 设置绘图的子图位置
plot_idx = 1
# 定义四个基分类器
models = [DecisionTreeClassifier(max_depth=None), # 决策树
RandomForestClassifier(n_estimators=n_estimators), # 随机森林
ExtraTreesClassifier(n_estimators=n_estimators), # 极端随机树
AdaBoostClassifier(DecisionTreeClassifier(max_depth=3), # AdaBoost
n_estimators=n_estimators)]
# 开始绘制四个基分类器的决策面
for pair in ([0, 1], [0, 2], [2, 3]):
for model in models:
# 从数据集中选取两个特征作为x轴和y轴
X = iris.data[:, pair]
y = iris.target
# 随机化样本,将数据集分成训练集和测试集
idx = np.arange(X.shape[0])
np.random.seed(RANDOM_SEED)
np.random.shuffle(idx)
X = X[idx]
y = y[idx]
half = int(X.shape[0] / 2)
X_train, X_test = X[:half], X[half:]
y_train, y_test = y[:half], y[half:]
# 训练基分类器
model.fit(X_train, y_train)
# 绘制训练集和测试集的散点图
plt.subplot(3, 4, plot_idx)
plt.tight_layout()
plt.scatter(X_train[:, 0], X_train[:, 1], c=y_train, cmap=cmap, edgecolor='k')
plt.scatter(X_test[:, 0], X_test[:, 1], c=y_test, cmap=cmap, alpha=0.6, edgecolor='k')
# 绘制决策面轮廓线
xx, yy = np.meshgrid(np.arange(X[:, 0].min() - 1, X[:, 0].max() + 1, plot_step),
np.arange(X[:, 1].min() - 1, X[:, 1].max() + 1, plot_step))
Z = model.predict(np.c_[xx.ravel(), yy.ravel()])
Z = Z.reshape(xx.shape)
cs = plt.contourf(xx, yy, Z, cmap=cmap, alpha=.5)
# 绘制分类器猜测的决策面轮廓线
xx_coarser, yy_coarser = np.meshgrid(np.arange(X[:, 0].min() - 1, X[:, 0].max() + 1, plot_step_coarser),
np.arange(X[:, 1].min() - 1, X[:, 1].max() + 1, plot_step_coarser))
Z_points_coarser = model.predict(np.c_[xx_coarser.ravel(), yy_coarser.ravel()]).reshape(xx_coarser.shape)
cs_points = plt.scatter(xx_coarser, yy_coarser, s=15, c=Z_points_coarser, cmap=cmap, edgecolor='none')
# 设置图像的标题和绘图的标签
plt.title(pair)
plot_idx += 1
# 显示绘制结果
plt.suptitle("Classifiers on feature subsets of the Iris dataset")
plt.axis("tight")
plt.show()
```
python 代码 使用随机森林分类模型对银行客户违约信息数据集进行预测,并对n_estimators和max_features两个参数进行调参,获得最优参数
在Python中,我们可以使用scikit-learn库中的RandomForestClassifier来进行银行客户违约预测。以下是基本步骤以及如何调整`n_estimators`和`max_features`这两个关键参数:
1. 首先,需要导入必要的库:
```python
import pandas as pd
from sklearn.model_selection import train_test_split, GridSearchCV
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score, classification_report
```
2. 加载并预处理数据集:
```python
data = pd.read_csv('bank_default.csv') # 假设数据文件名
X = data.drop('default', axis=1) # 特征变量
y = data['default'] # 目标变量
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
3. 定义参数网格(Grid Search)来寻找最佳的`n_estimators`和`max_features`:
```python
param_grid = {
'n_estimators': [50, 100, 200],
'max_features': ['auto', 'sqrt', 'log2']
}
```
4. 创建RandomForestClassifier实例并进行交叉验证:
```python
rfc = RandomForestClassifier(random_state=42)
grid_search = GridSearchCV(rfc, param_grid, cv=5, scoring='accuracy')
grid_search.fit(X_train, y_train)
```
5. 找到最佳参数组合:
```python
best_params = grid_search.best_params_
print(f"Best parameters found: {best_params}")
```
6. 使用最佳参数对测试集进行预测:
```python
optimized_rfc = RandomForestClassifier(**best_params, random_state=42)
optimized_rfc.fit(X_train, y_train)
y_pred = optimized_rfc.predict(X_test)
```
7. 评估模型性能:
```python
accuracy = accuracy_score(y_test, y_pred)
report = classification_report(y_test, y_pred)
print(f"Accuracy: {accuracy*100:.2f}%")
print("Classification Report:")
print(report)
```
阅读全文
相关推荐
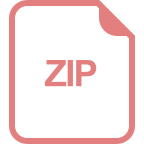
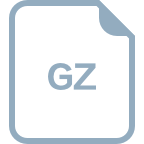
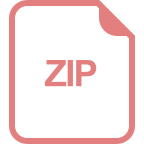
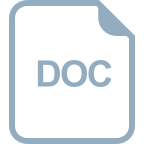












