防空火力资源调度Python代码 现已知武器种类和数量 目标种类和数量 目标突防概率
时间: 2024-10-11 11:15:40 浏览: 28
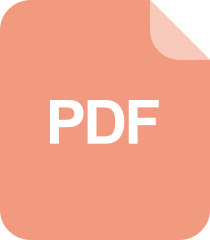
突防中火力/电子武器多目标攻击协同决策
防空火力资源调度是一个复杂的问题,涉及到多个因素。这里我假设你需要一个简化的模型来模拟武器和目标之间的分配。
首先,我们需要明确几个关键点:
1. 武器种类和数量
2. 目标种类和数量
3. 目标突防概率
在这个简化的模型中,我们可以使用贪心算法或线性规划等方法来进行资源调度。以下是一个简化的Python代码示例,使用贪心算法进行资源调度:
```python
# 定义武器类
class Weapon:
def __init__(self, name, quantity, effectiveness):
self.name = name
self.quantity = quantity
self.effectiveness = effectiveness # 有效率,即击中概率
# 定义目标类
class Target:
def __init__(self, name, quantity, penetration_probability):
self.name = name
self.quantity = quantity
self.penetration_probability = penetration_probability # 突防概率
# 资源调度函数
def schedule_resources(weapons, targets):
weapon_index = 0
target_index = 0
allocations = [] # 保存分配结果
while weapons[weapon_index].quantity > 0 and targets[target_index].quantity > 0:
if weapons[weapon_index].effectiveness >= targets[target_index].penetration_probability:
allocations.append((weapons[weapon_index].name, targets[target_index].name))
weapons[weapon_index].quantity -= 1
targets[target_index].quantity -= 1
else:
weapon_index += 1
if weapon_index >= len(weapons):
break
return allocations
# 示例数据
weapons = [
Weapon("WeaponA", 10, 0.9),
Weapon("WeaponB", 5, 0.8)
]
targets = [
Target("TargetX", 15, 0.7),
Target("TargetY", 10, 0.6)
]
# 执行调度
allocations = schedule_resources(weapons, targets)
print("资源调度结果:", allocations)
```
这个代码示例中,`schedule_resources` 函数会尝试将每一种武器分配给每一个目标,优先选择有效率高于目标突防概率的武器。如果所有武器都无法有效对付某个目标,则跳过该目标。
阅读全文
相关推荐
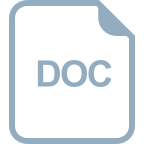
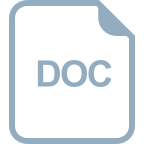
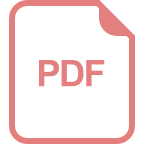
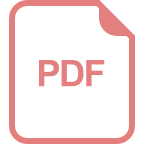
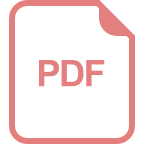
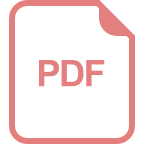
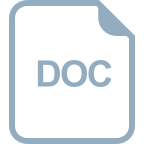
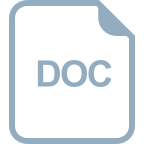
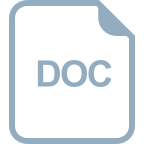
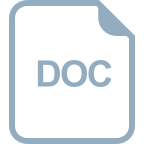
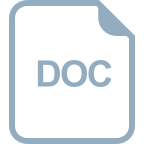
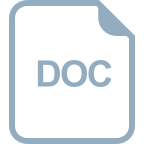
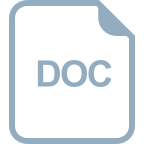


