pthread_exit abort
时间: 2023-08-13 12:06:11 浏览: 60
pthread_exit和abort是两个不同的函数,用于不同的目的。
1. pthread_exit:
pthread_exit函数用于在线程中终止当前线程的执行,并返回一个退出码。它会立即终止线程的执行,并释放线程所占用的资源。通常情况下,pthread_exit函数会在线程完成任务后被调用,将线程的执行结果传递给其他线程或进程。
2. abort:
abort函数用于终止程序的执行。当调用abort函数时,程序会立即终止并生成一个异常终止信号。这个信号通常会被操作系统捕获,并进行相应的处理。通常情况下,abort函数会在发生严重错误或异常情况时被调用,以避免程序运行进一步导致问题。
需要注意的是,pthread_exit只会终止当前线程的执行,而不会终止整个程序的执行。而abort函数会导致整个程序异常终止。
相关问题
pthread_exit
`pthread_exit`是一个函数调用,用于在线程执行完毕后终止线程。它可以在任何地方调用,包括线程的起点函数中。调用`pthread_exit`会立即终止当前线程的执行,并返回一个指定的退出状态。这个状态可以被其他线程使用,以便知道线程的终止原因或结果。
`pthread_exit`的函数原型如下:
```c
void pthread_exit(void *retval);
```
其中,`retval`参数是一个指向线程退出状态的指针。这个指针可以被其他线程使用,以便获取线程的退出状态信息。
注意,调用`pthread_exit`会终止当前线程,但不会影响其他正在运行的线程。如果主线程调用了`pthread_exit`,那么整个进程也会终止。
这就是关于`pthread_exit`函数的简要说明。如果你有其他问题,请随时提问。
pthread_cancel pthread_exit
pthread_exit和pthread_cancel都是用于线程的退出,但是它们的作用不同。pthread_exit用于当前线程退出,而pthread_cancel是用于取消另一个线程的执行。具体来说,pthread_exit会使当前线程立即退出,并返回一个指定的退出码,而pthread_cancel则是向指定的线程发送一个取消请求,如果该线程没有被设置为不可取消状态,那么它将会被取消。
pthread_cond_wait是一个线程同步的函数,它会使当前线程等待在一个条件变量上,直到另一个线程调用pthread_cond_signal或pthread_cond_broadcast函数来通知它。在等待之前,必须先获得一个互斥锁,以保证线程安全。
相关推荐
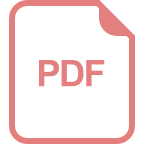












