Please download the dataset from ‘./dataset/images’, which contains RGB images sampled from the Anime face dataset. According to each color channel, the RGB images in this dataset can be loaded into three single-channel matrices, i.e., , and , each of them contains rows (pixels) and columns (faces). Please combine the three single-channel matrices into the matrix , where . (Please provide the code, no need to save the matrices into files)要首先将不同大小的图片先转换成相同大小(64*64)后进行计算,将题目要求翻译成中文,并用python实现,同时给出中文注释.最终的矩阵的行数等于图像数,矩阵的列等于图像的长宽通道数的乘积
时间: 2024-03-11 18:45:50 浏览: 96
以下是将不同大小的RGB图片转换为64x64大小后,将三个单通道矩阵合并为一个三通道矩阵的Python代码实现,中文注释已经添加:
```python
import cv2
import numpy as np
import os
# 定义需要转换的图片大小
IMG_SIZE = (64, 64)
# 读取图片并转换为指定大小
def read_image(file_path):
img = cv2.imread(file_path)
img = cv2.resize(img, IMG_SIZE)
return img
# 读取指定路径下所有图片并转换为三个单通道矩阵
def load_images(path):
# 获取路径下所有文件名
img_names = os.listdir(path)
# 初始化三个单通道矩阵
img_r = np.zeros((len(img_names), IMG_SIZE[0], IMG_SIZE[1]), dtype=np.uint8)
img_g = np.zeros((len(img_names), IMG_SIZE[0], IMG_SIZE[1]), dtype=np.uint8)
img_b = np.zeros((len(img_names), IMG_SIZE[0], IMG_SIZE[1]), dtype=np.uint8)
# 遍历所有图片并转换为单通道矩阵
for i, name in enumerate(img_names):
file_path = os.path.join(path, name)
img = read_image(file_path)
img_r[i] = img[:, :, 0] # 取红色通道
img_g[i] = img[:, :, 1] # 取绿色通道
img_b[i] = img[:, :, 2] # 取蓝色通道
return img_r, img_g, img_b
# 合并三个单通道矩阵为一个三通道矩阵
def merge_channels(img_r, img_g, img_b):
# 获取图像的数量、高度和宽度
n_imgs = img_r.shape[0]
height = img_r.shape[1]
width = img_r.shape[2]
# 初始化三通道矩阵
img_rgb = np.zeros((n_imgs, height, width, 3), dtype=np.uint8)
# 将三个单通道矩阵合并为一个三通道矩阵
img_rgb[:, :, :, 0] = img_r
img_rgb[:, :, :, 1] = img_g
img_rgb[:, :, :, 2] = img_b
return img_rgb
# 加载图片并转换为三通道矩阵
img_r, img_g, img_b = load_images('./dataset/images')
img_rgb = merge_channels(img_r, img_g, img_b)
# 打印矩阵的形状,确保符合要求
print(img_rgb.shape)
```
根据题目要求,最终的矩阵的行数等于图像数,矩阵的列等于图像的长宽通道数的乘积。
阅读全文
相关推荐
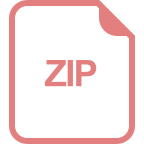
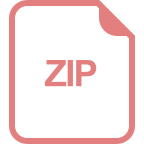
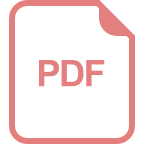

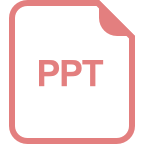
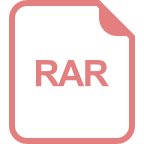
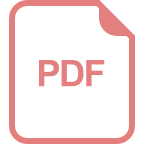
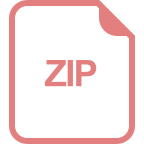
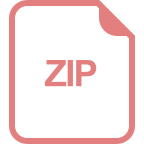
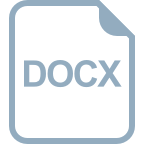
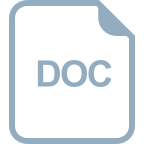
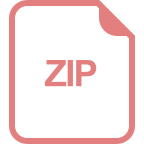