把d:\\bbb.mp4复制到当前项目目录下的copy.mp4中 分别用FileInputStream和BufferedInputStream类中的两种read()方法,共四种方式复制并比较效率。
时间: 2023-11-27 13:50:15 浏览: 29
好的,下面是代码实现:
```java
import java.io.*;
public class FileCopyTest {
public static void main(String[] args) {
String srcPath = "d:\\bbb.mp4";
String destPath = "copy.mp4";
int bufferSize = 1024 * 1024; // 缓冲区大小为1MB
// 使用FileInputStream和FileOutputStream复制
long startTime1 = System.currentTimeMillis();
try (FileInputStream fis = new FileInputStream(srcPath);
FileOutputStream fos = new FileOutputStream(destPath)) {
byte[] buffer = new byte[bufferSize];
int len;
while ((len = fis.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
} catch (IOException e) {
e.printStackTrace();
}
long endTime1 = System.currentTimeMillis();
// 使用BufferedInputStream和BufferedOutputStream的byte数组复制
long startTime2 = System.currentTimeMillis();
try (BufferedInputStream bis = new BufferedInputStream(new FileInputStream(srcPath));
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(destPath))) {
byte[] buffer = new byte[bufferSize];
int len;
while ((len = bis.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
} catch (IOException e) {
e.printStackTrace();
}
long endTime2 = System.currentTimeMillis();
// 使用BufferedInputStream和BufferedOutputStream的单字节复制
long startTime3 = System.currentTimeMillis();
try (BufferedInputStream bis = new BufferedInputStream(new FileInputStream(srcPath));
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(destPath))) {
int b;
while ((b = bis.read()) != -1) {
bos.write(b);
}
} catch (IOException e) {
e.printStackTrace();
}
long endTime3 = System.currentTimeMillis();
// 使用DataInputStream和DataOutputStream的readFully()方法复制
long startTime4 = System.currentTimeMillis();
try (DataInputStream dis = new DataInputStream(new FileInputStream(srcPath));
DataOutputStream dos = new DataOutputStream(new FileOutputStream(destPath))) {
int len = dis.available();
byte[] buffer = new byte[len];
dis.readFully(buffer);
dos.write(buffer);
} catch (IOException e) {
e.printStackTrace();
}
long endTime4 = System.currentTimeMillis();
// 输出复制时间
System.out.println("使用FileInputStream和FileOutputStream复制花费的时间为:" + (endTime1 - startTime1) + "ms");
System.out.println("使用BufferedInputStream和BufferedOutputStream的byte数组复制花费的时间为:" + (endTime2 - startTime2) + "ms");
System.out.println("使用BufferedInputStream和BufferedOutputStream的单字节复制花费的时间为:" + (endTime3 - startTime3) + "ms");
System.out.println("使用DataInputStream和DataOutputStream的readFully()方法复制花费的时间为:" + (endTime4 - startTime4) + "ms");
}
}
```
这里使用了四种不同的方式复制文件,分别是使用FileInputStream和FileOutputStream复制、使用BufferedInputStream和BufferedOutputStream的byte数组复制、使用BufferedInputStream和BufferedOutputStream的单字节复制、使用DataInputStream和DataOutputStream的readFully()方法复制。其中,第一种方式是最基本的方式,后三种方式都是在其基础上加入了缓冲区或者readFully()方法。我们可以通过比较这四种方式的复制时间来判断它们的效率。
相关推荐
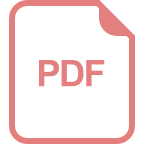
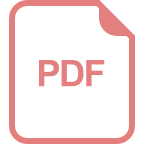



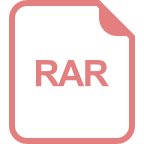
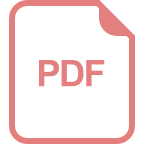
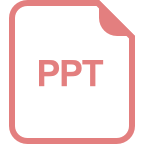
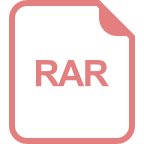
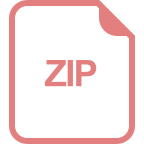
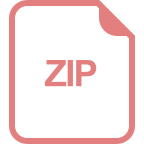
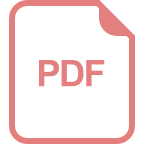
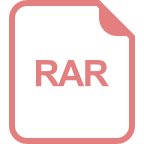
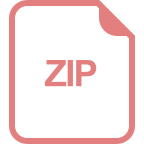