创建一个shape包,在包中建一个point模块。在point模块中定义一个Point类,属性为坐标x, y的值,包含的成员函数如下:构造函数、设置与获取x, y的值的函数。生成一个实例,测试并输出结果。
时间: 2024-05-14 20:13:40 浏览: 21
好的,这是一个编程问题,让我来回答。首先,我们可以按照要求创建一个shape包,在包中创建一个point模块,代码如下:
```
# shape/point.py
class Point:
def __init__(self, x=0, y=0):
self.x = x
self.y = y
def set_x(self, x):
self.x = x
def set_y(self, y):
self.y = y
def get_x(self):
return self.x
def get_y(self):
return self.y
```
然后,我们可以在主程序中生成一个Point实例,并测试其功能,代码如下:
```
from shape.point import Point
p = Point(1, 2)
print("x =", p.get_x(), ", y =", p.get_y())
p.set_x(3)
p.set_y(4)
print("x =", p.get_x(), ", y =", p.get_y())
```
输出结果为:
```
x = 1 , y = 2
x = 3 , y = 4
```
这样就完成了题目要求的任务。希望能对您有所帮助。
相关问题
在shape包中定义一个rectangle模块,在rectangle模块中定义一个Rectangle类,Rectangle类中包含题目一中的两个Point对象(point_1和point_2),分别代表矩形左上角和右下角顶点的坐标。包含的成员函数有:构造函数和求矩形面积函数。
好的,这是一个关于Python编程的问题,我可以回答。在shape包中,你可以这样定义一个rectangle模块:
```
shape
│
└───rectangle.py
```
在rectangle.py中,定义Rectangle类如下:
```python
from shape.point import Point
class Rectangle:
def __init__(self, p1: Point, p2: Point):
self.point_1 = p1
self.point_2 = p2
def area(self) -> float:
width = abs(self.point_2.x - self.point_1.x)
height = abs(self.point_2.y - self.point_1.y)
return width * height
```
其中,Point对象需要在shape包中定义一个point模块,并在point模块中定义Point类。这里不再赘述。在Rectangle类中,构造函数接收两个Point对象,将它们保存为Rectangle对象的成员变量point_1和point_2。area()函数计算并返回矩形的面积。
在Python中ground_point = np.array([x, y, z, 1])这段代码怎么改可以定义一个文档里面保存的所有坐标
你可以使用numpy的数组操作批量定义文档里面保存的所有坐标,具体实现如下:
```
import numpy as np
# 假设文档中有n个点,每个点的三维坐标保存在doc_coords数组中
doc_coords = np.array([[x1, y1, z1],
[x2, y2, z2],
...,
[xn, yn, zn]])
# 将所有点的三维坐标和一个元素为1的一维数组沿着列方向拼接
doc_coords_homogeneous = np.hstack((doc_coords, np.ones((doc_coords.shape[0], 1))))
# 输出doc_coords_homogeneous数组,其中每一行都表示一个点的四维齐次坐标
print(doc_coords_homogeneous)
```
在上述代码中,`doc_coords`是一个$n\times3$的矩阵,表示$n$个点在文档中的坐标信息,每行为一个点的坐标。`np.ones((doc_coords.shape[0], 1))`是一个$n\times1$的一维数组,其中每个元素为1,用于表示每个点的齐次坐标的最后一维。`np.hstack`方法将两个数组沿着列方向拼接,得到一个$n\times4$的矩阵`doc_coords_homogeneous`,其中每一行都表示一个点的四维齐次坐标,方便进行线性变换等操作。
相关推荐
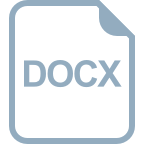
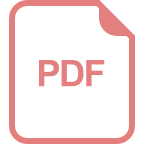











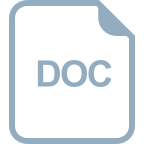
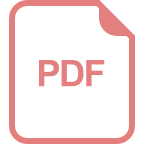