用c语言写一个具有对局回放功能的五子棋小游戏
时间: 2024-03-23 08:43:29 浏览: 15
好的,下面是一个简单的五子棋小游戏的代码,它具有对局回放功能,使用 ANSI C 语言编写,可以在命令行窗口运行。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define BOARD_SIZE 15
#define TRUE 1
#define FALSE 0
/* 棋盘 */
char board[BOARD_SIZE][BOARD_SIZE];
/* 落子位置 */
int x, y;
/* 当前玩家 */
char player;
/* 历史记录栈 */
int history[BOARD_SIZE * BOARD_SIZE][2];
int top = -1;
/* 打印棋盘 */
void print_board()
{
int i, j;
printf(" ");
for (i = 0; i < BOARD_SIZE; i++)
printf("%d ", i);
printf("\n");
for (i = 0; i < BOARD_SIZE; i++)
{
printf("%d ", i);
for (j = 0; j < BOARD_SIZE; j++)
{
printf("%c ", board[i][j]);
}
printf("\n");
}
}
/* 判断是否胜利 */
int check_win(int x, int y)
{
int i, j, count;
char chessman = board[x][y];
/* 水平方向 */
count = 1;
for (i = x - 1; i >= 0 && board[i][y] == chessman; i--)
count++;
for (i = x + 1; i < BOARD_SIZE && board[i][y] == chessman; i++)
count++;
if (count >= 5)
return TRUE;
/* 竖直方向 */
count = 1;
for (j = y - 1; j >= 0 && board[x][j] == chessman; j--)
count++;
for (j = y + 1; j < BOARD_SIZE && board[x][j] == chessman; j++)
count++;
if (count >= 5)
return TRUE;
/* 左上到右下方向 */
count = 1;
for (i = x - 1, j = y - 1; i >= 0 && j >= 0 && board[i][j] == chessman; i--, j--)
count++;
for (i = x + 1, j = y + 1; i < BOARD_SIZE && j < BOARD_SIZE && board[i][j] == chessman; i++, j++)
count++;
if (count >= 5)
return TRUE;
/* 右上到左下方向 */
count = 1;
for (i = x - 1, j = y + 1; i >= 0 && j < BOARD_SIZE && board[i][j] == chessman; i--, j++)
count++;
for (i = x + 1, j = y - 1; i < BOARD_SIZE && j >= 0 && board[i][j] == chessman; i++, j--)
count++;
if (count >= 5)
return TRUE;
return FALSE;
}
/* 保存历史记录 */
void save_history(int x, int y)
{
top++;
history[top][0] = x;
history[top][1] = y;
}
/* 悔棋 */
void undo()
{
if (top >= 0)
{
board[history[top][0]][history[top][1]] = '+';
top--;
}
print_board();
}
/* 对弈 */
void play_game()
{
int i, j;
int flag = 0;
srand((unsigned int)time(NULL));
/* 随机先手 */
if (rand() % 2 == 0)
{
printf("电脑先手\n");
player = 'O';
/* 电脑落子 */
x = rand() % BOARD_SIZE;
y = rand() % BOARD_SIZE;
board[x][y] = player;
save_history(x, y);
print_board();
}
else
{
printf("玩家先手\n");
player = 'X';
print_board();
}
while (1)
{
if (player == 'X')
{
printf("请玩家输入下棋的位置(x y):");
scanf("%d %d", &x, &y);
if (x < 0 || x >= BOARD_SIZE || y < 0 || y >= BOARD_SIZE || board[x][y] != '+')
{
printf("无效位置,请重新输入\n");
continue;
}
board[x][y] = player;
save_history(x, y);
}
else
{
printf("电脑落子:");
/* 简单的电脑AI,随机选择 */
while (1)
{
x = rand() % BOARD_SIZE;
y = rand() % BOARD_SIZE;
if (board[x][y] == '+')
break;
}
board[x][y] = player;
save_history(x, y);
}
print_board();
if (check_win(x, y))
{
if (player == 'X')
printf("玩家胜利!\n");
else
printf("电脑胜利!\n");
flag = 1;
break;
}
if (top == BOARD_SIZE * BOARD_SIZE - 1)
{
printf("平局!\n");
flag = 1;
break;
}
player = (player == 'X') ? 'O' : 'X';
}
/* 对局结束,询问是否回放 */
printf("是否回放对局记录?(Y/N)");
char c;
scanf("%c", &c);
if (c == 'Y' || c == 'y')
{
printf("对局回放:\n");
for (i = 0; i <= top; i++)
{
printf("%c (%d,%d)\n", (i % 2 == 0) ? 'X' : 'O', history[i][0], history[i][1]);
}
}
}
int main()
{
int i, j;
/* 初始化棋盘 */
for (i = 0; i < BOARD_SIZE; i++)
for (j = 0; j < BOARD_SIZE; j++)
board[i][j] = '+';
play_game();
return 0;
}
```
这个程序中,使用了一个二维字符数组来表示棋盘,字符 '+' 表示空位置,字符 'X' 和 'O' 分别表示玩家和电脑的棋子。玩家和电脑轮流下棋,当有一方胜利或者棋盘填满时,游戏结束。当游戏结束后,程序会询问是否回放对局记录,如果选择回放,则会打印出历史记录。
相关推荐
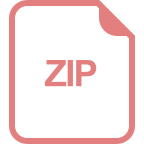
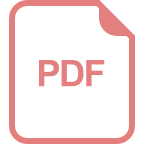
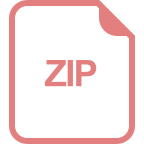









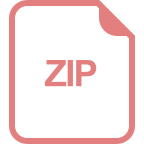
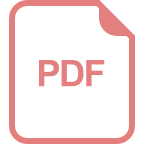
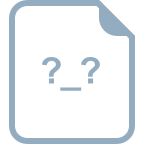
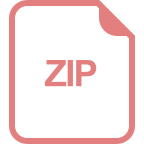