用C语言写一段代码实现推箱子小游戏
时间: 2023-12-29 11:45:32 浏览: 85
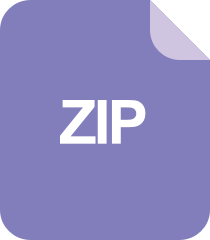
C语言写的推箱子小游戏.zip
推箱子小游戏是一种经典的益智游戏,可以用C语言实现。以下是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h> // 需要用到 getch() 函数
#define ROW 9 // 地图行数
#define COL 9 // 地图列数
#define WALL '#' // 墙
#define BOX '$' // 箱子
#define TARGET '.' // 目标位置
#define MAN '@' // 人物
char map[ROW][COL + 1] = { // 地图
"#########",
"# # #",
"# $ #",
"# . #",
"# @ #",
"# . #",
"# $ #",
"# # #",
"#########"
};
int man_x = 4; // 人物初始位置
int man_y = 4;
int check(int x, int y, int dx, int dy) // 检查是否可以移动
{
if (map[x + dx][y + dy] == WALL) return 0; // 碰到墙
if (map[x + dx][y + dy] == BOX) // 箱子
{
if (map[x + dx * 2][y + dy * 2] == WALL || map[x + dx * 2][y + dy * 2] == BOX) return 0; // 碰到墙或箱子
map[x + dx][y + dy] = ' ';
map[x + dx * 2][y + dy * 2] = BOX;
}
return 1;
}
void display() // 显示地图
{
system("cls"); // 清屏
for (int i = 0; i < ROW; i++)
{
printf("%s\n", map[i]);
}
}
int main()
{
char ch;
display();
while (1)
{
ch = getch(); // 获取键盘输入
switch (ch)
{
case 'W': // 上移
case 'w':
if (check(man_x, man_y, -1, 0))
{
map[man_x][man_y] = ' ';
man_x--;
map[man_x][man_y] = MAN;
}
break;
case 'S': // 下移
case 's':
if (check(man_x, man_y, 1, 0))
{
map[man_x][man_y] = ' ';
man_x++;
map[man_x][man_y] = MAN;
}
break;
case 'A': // 左移
case 'a':
if (check(man_x, man_y, 0, -1))
{
map[man_x][man_y] = ' ';
man_y--;
map[man_x][man_y] = MAN;
}
break;
case 'D': // 右移
case 'd':
if (check(man_x, man_y, 0, 1))
{
map[man_x][man_y] = ' ';
man_y++;
map[man_x][man_y] = MAN;
}
break;
default:
break;
}
display();
}
return 0;
}
```
这个程序中,地图用二维字符数组 `map` 表示,人物的位置用 `man_x` 和 `man_y` 表示,通过 `getch()` 函数获取键盘输入,然后根据键盘输入移动人物。其中 `check()` 函数用来检查是否可以移动,如果可以,则将人物移动到新位置,并且如果有箱子,还需要将箱子也一起移动。最后调用 `display()` 函数显示地图。
阅读全文
相关推荐
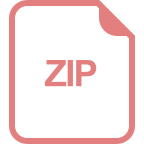
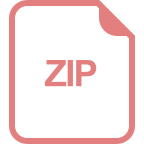















