快速行进路径规划(FM)算法
时间: 2024-12-29 20:13:52 浏览: 9
### Fast Marching (FM) Algorithm for Path Planning
The Fast Marching (FM) algorithm offers a robust approach to path planning within the realms of computer science and robotics. This method defines a speed map over which robots can navigate, simulating wave propagation from start points outward until all reachable areas are covered or obstacles halt further expansion[^1]. Each point on this map receives an arrival time based upon when the simulated wave front reaches it.
For each location in space, computing its minimum travel duration from any other position becomes feasible once such times have been established across the entire domain. By tracing back along decreasing values starting at destination coordinates towards origin ones, one obtains paths characterized by minimal traversal durations—this constitutes the core concept behind applying FM techniques during route determination tasks.
Compared against alternative potential field-based strategies, FM avoids issues related to local minima traps while ensuring completeness alongside consistency as demonstrated theoretically elsewhere. Performance-wise, both A* search algorithms share comparable computational complexities expressed mathematically as O(N log N), where N represents total nodes processed throughout execution cycles.
In practical applications like quadrotor navigation systems, integrating online motion planners capable of rapidly adapting velocity fields allows drones not only to discover optimal trajectories but also expand flight corridors dynamically according to environmental conditions encountered en route[^2]. Furthermore, leveraging optimization methods ensures generated Bernstein polynomial curves adhere strictly to safety requirements besides maintaining dynamic feasibility constraints necessary for real-world deployment scenarios involving autonomous aerial vehicles operating under varying circumstances without compromising performance standards expected within industry contexts today.
```python
import numpy as np
from scipy.interpolate import RegularGridInterpolator
def fast_marching_method(grid_size=100, obstacle_positions=None):
"""
Demonstrates basic principles underlying Fast Marching Method.
Parameters:
grid_size : int
Size of square grid representing environment dimensions.
obstacle_positions : list[tuple], optional
List containing tuples indicating positions occupied by static objects.
Returns:
tuple[np.ndarray, float]
Tuple consisting of computed distance transform array followed by maximum finite value found therein.
"""
# Initialize empty grids for tracking distances & visited status per cell
dt = np.full((grid_size,)*2, fill_value=np.inf)
open_set = set()
# Define source node located centrally within defined bounds
src_x, src_y = grid_size//2, grid_size//2
# Assign zero cost-to-come initially assigned solely thereat
dt[src_x][src_y] = 0.0
open_set.add((src_x, src_y))
def neighbors(x, y):
"""Yield valid neighboring cells around given coordinate pair."""
yield from ((nx, ny) for nx, ny in [
(x-1,y),(x+1,y),
(x ,y-1),(x ,y+1)]
if 0<=nx<grid_size and 0<=ny<grid_size)
while len(open_set)>0:
current_node = min(open_set,key=lambda p:dt[p])
cx,cy=current_node
del open_set
for neighbor in filter(lambda n:n not in closed_set,
neighbors(cx,cy)):
tentative_gscore = (
dt[cx][cy]+np.sqrt(
abs(neighbor[0]-cx)**2+
abs(neighbor[1]-cy)**2))
if tentative_gscore < dt[neighbor]:
dt[neighbor]=tentative_gscore
if neighbor not in open_set.union(closed_set):
open_set|={neighbor}
max_finite_dt_val=max(filter(np.isfinite,dt.flatten()))
return dt,max_finite_dt_val
if __name__=='__main__':
_,max_dist=fast_marching_method(obstacle_positions=[(i,j)for i in range(35,65)for j in [34]])
print(f'Maximum Distance Value Found:{round(max_dist,ndigits=2)}')
```
阅读全文
相关推荐








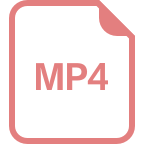







