用CLion建立一个线性表,依次输入数据元素1,2,3,4,5,6,7,8,9,10,删除数据元素5,依次显示当前线性表中的数据元素,实现ListSearch函数,并输出该线性表中依次搜索9和11时得到的索值
时间: 2024-09-27 07:06:43 浏览: 34
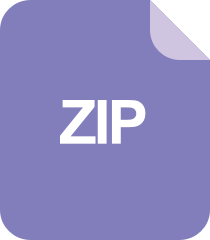
数据结构2-1章节线性表源码
在CLion中创建一个简单的线性表(通常可以是一个动态数组或者链表),首先需要定义一个包含数据元素的数据结构,比如`ListNode`(对于链表)或者`Element`(数组)。这里我们假设使用链表作为例子:
```cpp
// ListNode.h
class ListNode {
public:
int data;
ListNode* next;
ListNode(int value) : data(value), next(nullptr) {}
};
```
然后你需要实现一个双向链表,包括插入、删除和搜索功能:
```cpp
// List.cpp
#include "ListNode.h"
void insertToList(ListNode*& head, int value) {
if (head == nullptr) {
head = new ListNode(value);
} else {
ListNode* newNode = new ListNode(value);
newNode->next = head;
head = newNode;
}
}
void deleteFromList(ListNode*& head, int value) {
if (head == nullptr || head->data != value) return;
ListNode* temp = head;
head = head->next;
delete temp;
}
int listSearch(const ListNode* head, int value) {
ListNode* current = head;
while (current != nullptr) {
if (current->data == value) return current - head; // 返回元素在列表中的位置
current = current->next;
}
return -1; // 如果未找到,返回-1
}
```
接下来,在主程序中,你可以按照描述一步步操作:
```cpp
int main() {
ListNode* head = nullptr;
for (int i = 1; i <= 10; ++i) {
insertToList(head, i);
}
deleteFromList(head, 5);
// 显示并打印链表
ListNode* curr = head;
std::cout << "After deletion, the elements are: ";
while (curr != nullptr) {
std::cout << curr->data << " ";
curr = curr->next;
}
std::cout << "\n";
// 搜索并输出索引
int index9 = listSearch(head, 9);
int index11 = listSearch(head, 11); // 这里可能会返回-1,因为11不在列表中
std::cout << "Index of 9 is: " << index9 << "\n";
std::cout << "Index of 11 is: " << index11 << "\n";
return 0;
}
```
阅读全文
相关推荐
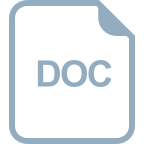
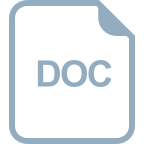
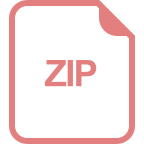
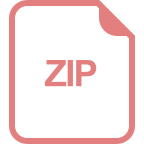
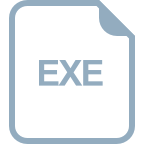










