dbn做信号分类tensorflow代码
时间: 2023-07-28 14:03:47 浏览: 101
DBN(深度信念网络)是一种无监督学习算法,常用于处理高维数据的特征提取和分类任务。下面是使用TensorFlow实现DBN进行信号分类的示例代码:
```python
import tensorflow as tf
from tensorflow.contrib import learn
# 加载数据集
data = learn.datasets.load_dataset('your_dataset') # 替换为你的数据集
# 数据预处理
x_train = data.train.images
y_train = data.train.labels
x_test = data.test.images
y_test = data.test.labels
# 构建DBN模型
n_visible = x_train.shape[1] # 输入层节点数(特征数)
n_hidden = 300 # 隐层节点数(可以根据具体任务进行调整)
# 定义可见层和隐层
x = tf.placeholder("float", [None, n_visible]) # 可见层节点
W = tf.placeholder("float", [n_visible, n_hidden]) # 隐层权重
b_visible = tf.placeholder("float", [n_visible]) # 可见层偏置
b_hidden = tf.placeholder("float", [n_hidden]) # 隐层偏置
# DBN前向传播
def propup(layer, W, b):
return tf.nn.sigmoid(tf.matmul(layer, W) + b)
# DBN反向传播
def propdown(layer, W, b):
return tf.nn.sigmoid(tf.matmul(layer, tf.transpose(W)) + b)
# DBN重构
def sample_h_given_v(v0_sample):
h0_mean = propup(v0_sample, W, b_hidden)
h0_sample = tf.nn.relu(tf.sign(h0_mean - tf.random_uniform(tf.shape(h0_mean)))) # 随机采样
return h0_mean, h0_sample
def sample_v_given_h(h0_sample):
v1_mean = propdown(h0_sample, W, b_visible)
v1_sample = tf.nn.relu(tf.sign(v1_mean - tf.random_uniform(tf.shape(v1_mean)))) # 随机采样
return v1_mean, v1_sample
# 训练DBN
h0_mean, h0_sample = sample_h_given_v(x)
v1_mean, v1_sample = sample_v_given_h(h0_sample)
h1_mean, h1_sample = sample_h_given_v(v1_sample)
learning_rate = 0.1 # 学习率
k = 1 # CD-k采样次数
W_update = W + learning_rate * (tf.matmul(tf.transpose(x), h0_mean) - tf.matmul(tf.transpose(v1_sample), h1_mean))
b_visible_update = b_visible + learning_rate * tf.reduce_mean(x - v1_sample, 0)
b_hidden_update = b_hidden + learning_rate * tf.reduce_mean(h0_mean - h1_mean, 0)
updt = [W.assign(W_update), b_visible.assign(b_visible_update), b_hidden.assign(b_hidden_update)]
# 创建session并初始化变量
sess = tf.Session()
init = tf.global_variables_initializer()
sess.run(init)
# 执行CD-k过程
for epoch in range(10): # 迭代训练10次
for start, end in zip(range(0, len(x_train), 128), range(128, len(x_train), 128)):
batch = x_train[start:end]
sess.run(updt, feed_dict={x: batch})
# 使用DBN进行信号分类
h0, _ = sample_h_given_v(x_test)
predicted_labels = tf.argmax(h0, axis=1)
actual_labels = tf.argmax(y_test, axis=1)
accuracy = tf.reduce_mean(tf.cast(tf.equal(predicted_labels, actual_labels), tf.float32))
print("准确率:", sess.run(accuracy))
```
需要替换代码中的`your_dataset`为真实的数据集名称,并根据具体任务调整参数。以上代码使用DBN进行特征提取,并通过隐层的输出进行信号分类,最后输出分类准确率。
阅读全文
相关推荐
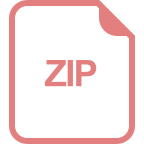
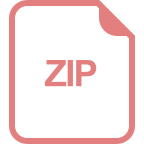
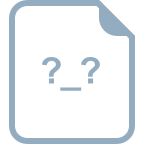
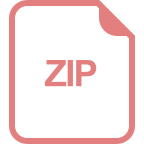
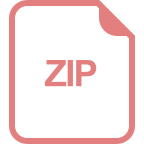
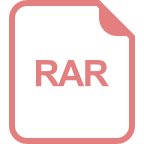
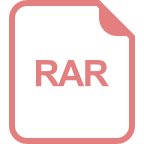
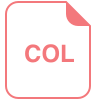
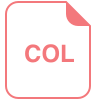
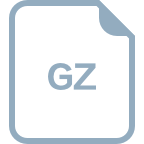
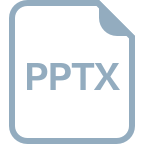
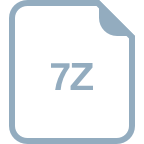
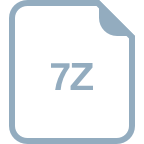