爬取任一城市的天气预报数据,并把爬取到的天气数据写入本地文本,并进行数据可视化分析python
时间: 2024-09-25 22:08:05 浏览: 47
在Python中,你可以使用requests库来抓取网页数据,BeautifulSoup或Scrapy等工具进行HTML解析,然后使用pandas处理和存储数据,最后通过matplotlib或seaborn库进行数据可视化。以下是基本步骤:
1. **安装必要的库**:
```
pip install requests beautifulsoup4 pandas matplotlib seaborn
```
2. **编写爬虫函数**:
- 首先,获取你要爬取的城市的天气预报网站URL,比如北京的天气可以去中国气象局官网或其他提供API的天气服务网站。
```python
import requests
from bs4 import BeautifulSoup
def get_weather_data(city):
url = "http://your_city_weather_url.com/" + city
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 解析页面提取天气数据,这里只是一个示例,实际需要根据网站结构调整
data = {} # 例如 {'date': ..., 'temperature': ...}
return data
```
3. **数据处理并写入文本文件**:
```python
def save_to_text(data, filename):
with open(filename, 'w', newline='', encoding='utf-8') as f:
for key, value in data.items():
f.write(f"{key}: {value}\n")
data = get_weather_data("北京")
save_to_text(data, "weather_data.txt")
```
4. **数据可视化**:
```python
import pandas as pd
df = pd.read_csv('weather_data.txt', sep=":", names=['Date', 'Temperature'])
plt.figure(figsize=(10,6))
df['Temperature'].plot(kind='line')
plt.title("北京天气温度变化")
plt.xlabel('日期')
plt.ylabel('温度')
plt.show()
```
阅读全文
相关推荐
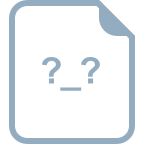
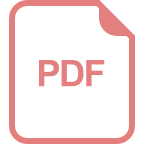

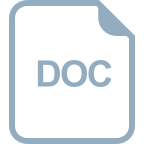
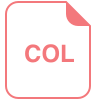
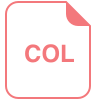
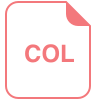
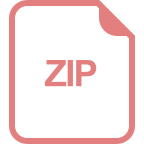
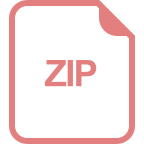
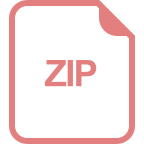
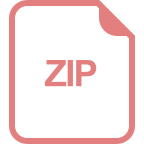
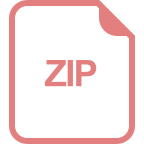
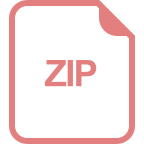