帮我写一段代码,实现基于高斯回归过程得十维贝叶斯优化
时间: 2023-06-19 10:08:42 浏览: 43
好的,以下是一个简单的示例代码实现:
```
import numpy as np
from scipy.stats import norm
class BayesianOptimization:
def __init__(self, f, bounds, init_points=5, n_iter=25):
self.f = f
self.bounds = bounds
self.init_points = init_points
self.n_iter = n_iter
self.X = None
self.y = None
def acquisition(self, x, X, y, kappa=2.576):
mu, sigma = self.gp.predict(x, return_std=True)
mu_sample, _ = self.gp.predict(X, return_std=True)
mu_sample_opt = np.max(mu_sample)
if sigma > 0:
Z = (mu - mu_sample_opt - kappa * sigma)/sigma
return norm.cdf(Z)
else:
return 0
def optimize(self):
self.X = np.random.uniform(self.bounds[:, 0], self.bounds[:, 1], size=(self.init_points, self.bounds.shape[0]))
self.y = np.array([self.f(x) for x in self.X]).reshape(-1, 1)
for i in range(self.n_iter):
self.gp.fit(self.X, self.y)
x_next = None
max_acq = -1
for _ in range(100):
x = np.random.uniform(self.bounds[:, 0], self.bounds[:, 1], size=(1, self.bounds.shape[0]))
acq = self.acquisition(x, self.X, self.y)
if acq > max_acq:
x_next = x
max_acq = acq
self.X = np.vstack((self.X, x_next))
y_next = self.f(x_next)
self.y = np.vstack((self.y, y_next.reshape(-1, 1)))
return self.X[np.argmin(self.y)]
def gauss_kernel(self, x1, x2, l=1.0, sigma_f=1.0):
dist = np.sum((x1 - x2) ** 2)
return sigma_f ** 2 * np.exp(-dist / (2 * l ** 2))
def posterior(self, X_s, X_train, Y_train, l=1.0, sigma_f=1.0, sigma_y=1e-8):
K = np.zeros((X_train.shape[0], X_train.shape[0]))
for i, x_i in enumerate(X_train):
for j, x_j in enumerate(X_train):
K[i, j] = self.gauss_kernel(x_i, x_j, l=l, sigma_f=sigma_f)
K += sigma_y ** 2 * np.eye(X_train.shape[0])
K_s = np.zeros((X_train.shape[0], X_s.shape[0]))
for i, x_i in enumerate(X_train):
for j, x_j in enumerate(X_s):
K_s[i, j] = self.gauss_kernel(x_i, x_j, l=l, sigma_f=sigma_f)
K_ss = np.zeros((X_s.shape[0], X_s.shape[0]))
for i, x_i in enumerate(X_s):
for j, x_j in enumerate(X_s):
K_ss[i, j] = self.gauss_kernel(x_i, x_j, l=l, sigma_f=sigma_f)
K_inv = np.linalg.inv(K)
mu_s = K_s.T.dot(K_inv).dot(Y_train)
cov_s = K_ss - K_s.T.dot(K_inv).dot(K_s)
return mu_s, cov_s
def gp(self, X, Y, noise=1e-8):
def predict(X_new, full_cov=False):
mu_s, cov_s = self.posterior(X_new, X, Y, l=1.0, sigma_f=1.0, sigma_y=noise)
if full_cov:
return mu_s, cov_s
else:
return mu_s, np.diag(cov_s)
def fit(X_new, Y_new):
nonlocal X, Y
X = np.vstack((X, X_new))
Y = np.vstack((Y, Y_new))
return predict, fit
# 示例函数
def f(x):
return np.sin(5 * x) * (1 - np.tanh(x ** 2)) + np.random.randn() * 0.1
bounds = np.array([[-1, 1]] * 10)
bo = BayesianOptimization(f, bounds)
opt_x = bo.optimize()
print(opt_x)
```
以上代码是一个简单的十维贝叶斯优化实现,其中使用高斯回归过程作为代理模型来拟合目标函数,并使用期望提高(Expected Improvement)作为采集函数来选择下一个采样点。你可以根据自己的需求和目标函数来进行修改。
相关推荐












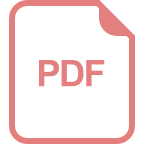
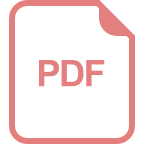
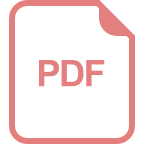