用C语言写函数分别实现:顺序查找、折半查找、二叉排序树查找、哈希查找,并在主函数生成一组随机数,或者输入一组数,然后输入查找数据,调用查找算法
时间: 2024-02-05 14:12:42 浏览: 75
顺序查找:
```c
#include <stdio.h>
int search(int arr[], int n, int x) {
for (int i = 0; i < n; i++) {
if (arr[i] == x) {
return i;
}
}
return -1;
}
int main() {
int arr[] = {9, 3, 5, 7, 1};
int n = sizeof(arr) / sizeof(arr[0]);
int x = 7;
int index = search(arr, n, x);
if (index == -1) {
printf("%d not found\n", x);
} else {
printf("%d found at index %d\n", x, index);
}
return 0;
}
```
折半查找:
```c
#include <stdio.h>
int binary_search(int arr[], int n, int x) {
int l = 0, r = n - 1;
while (l <= r) {
int mid = (l + r) / 2;
if (arr[mid] == x) {
return mid;
} else if (arr[mid] < x) {
l = mid + 1;
} else {
r = mid - 1;
}
}
return -1;
}
int main() {
int arr[] = {1, 3, 5, 7, 9};
int n = sizeof(arr) / sizeof(arr[0]);
int x = 7;
int index = binary_search(arr, n, x);
if (index == -1) {
printf("%d not found\n", x);
} else {
printf("%d found at index %d\n", x, index);
}
return 0;
}
```
二叉排序树查找:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
TreeNode *insert(TreeNode *root, int val) {
if (root == NULL) {
TreeNode *node = (TreeNode *)malloc(sizeof(TreeNode));
node->val = val;
node->left = NULL;
node->right = NULL;
return node;
}
if (val < root->val) {
root->left = insert(root->left, val);
} else if (val > root->val) {
root->right = insert(root->right, val);
}
return root;
}
int search(TreeNode *root, int val) {
if (root == NULL || root->val == val) {
return root != NULL;
}
if (val < root->val) {
return search(root->left, val);
} else {
return search(root->right, val);
}
}
int main() {
int arr[] = {9, 3, 5, 7, 1};
int n = sizeof(arr) / sizeof(arr[0]);
TreeNode *root = NULL;
for (int i = 0; i < n; i++) {
root = insert(root, arr[i]);
}
int x = 7;
if (search(root, x)) {
printf("%d found\n", x);
} else {
printf("%d not found\n", x);
}
return 0;
}
```
哈希查找:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct ListNode {
int val;
struct ListNode *next;
} ListNode;
typedef struct HashTable {
ListNode **arr;
int size;
} HashTable;
HashTable *createHashTable(int size) {
HashTable *hashTable = (HashTable *)malloc(sizeof(HashTable));
hashTable->arr = (ListNode **)calloc(size, sizeof(ListNode *));
hashTable->size = size;
return hashTable;
}
int hash(int key, int size) {
return key % size;
}
void insert(HashTable *hashTable, int key, int val) {
int h = hash(key, hashTable->size);
ListNode *node = (ListNode *)malloc(sizeof(ListNode));
node->val = val;
node->next = hashTable->arr[h];
hashTable->arr[h] = node;
}
int search(HashTable *hashTable, int key) {
int h = hash(key, hashTable->size);
ListNode *p = hashTable->arr[h];
while (p) {
if (p->val == key) {
return 1;
}
p = p->next;
}
return 0;
}
int main() {
int arr[] = {9, 3, 5, 7, 1};
int n = sizeof(arr) / sizeof(arr[0]);
HashTable *hashTable = createHashTable(n);
for (int i = 0; i < n; i++) {
insert(hashTable, arr[i], i);
}
int x = 7;
if (search(hashTable, x)) {
printf("%d found\n", x);
} else {
printf("%d not found\n", x);
}
return 0;
}
```
阅读全文
相关推荐
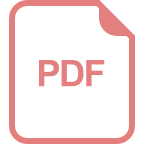
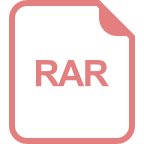
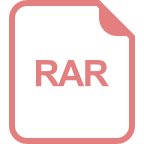















