用c语言写本关任务:从键盘输入一个字符串,用选择法对该字符串中的字符由小到大进行排序,并输出结果。
时间: 2024-02-12 11:02:21 浏览: 78
好的,这里是用选择排序算法来对字符串进行排序的C语言代码:
```
#include <stdio.h>
#include <string.h>
#define MAX_LENGTH 100
void selection_sort(char str[], int n);
int main()
{
char str[MAX_LENGTH];
printf("请输入字符串:\n");
fgets(str, MAX_LENGTH, stdin);
str[strcspn(str, "\n")] = '\0'; // 去除fgets自动添加的换行符
int n = strlen(str);
selection_sort(str, n);
printf("按字典序从小到大排序后的结果为:\n%s\n", str);
return 0;
}
void selection_sort(char str[], int n)
{
int i, j, min_idx;
for (i = 0; i < n - 1; i++)
{
min_idx = i;
for (j = i + 1; j < n; j++)
{
if (str[j] < str[min_idx])
{
min_idx = j;
}
}
char temp = str[min_idx];
str[min_idx] = str[i];
str[i] = temp;
}
}
```
在main()函数中,先通过fgets()函数从键盘输入字符串,然后去除自动添加的换行符。接着,计算字符串的长度n,然后调用selection_sort()函数对字符串进行排序。最后输出排序后的结果。
selection_sort()函数实现了选择排序的算法,每次选出未排序部分中的最小字符,放到已排序部分的末尾。
阅读全文
相关推荐
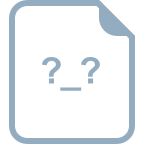















