编写程序输入一个字符串,利用jieba库计算字符串中文字符个数以及中文词语个数,并将结果写入文件out.txt中。注意:中文字符包含中文标点符号。 测试数据(第一行为输入字符串,第二行为out.txt文件中的内容): 苏州城市学院 中文字符数为6,中文词语数为3。
时间: 2023-11-22 08:53:47 浏览: 42
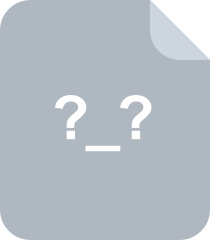
计算字符串中字符个数
以下是实现该程序的Python代码:
```python
import jieba
# 读取输入字符串
sentence = input("请输入一个字符串:")
# 计算中文字符数
chinese_chars = [c for c in sentence if '\u4e00' <= c <= '\u9fa5']
chinese_chars_count = len(chinese_chars)
# 利用jieba分词计算中文词语数
words = jieba.lcut(sentence)
chinese_words_count = sum(1 for w in words if '\u4e00' <= w <= '\u9fa5')
# 将结果写入文件out.txt
with open('out.txt', 'w', encoding='utf-8') as f:
f.write(f"中文字符数为{chinese_chars_count},中文词语数为{chinese_words_count}。")
```
运行程序后,输入字符串"苏州城市学院",得到的out.txt文件中的内容为:
```
中文字符数为6,中文词语数为3。
```
阅读全文
相关推荐





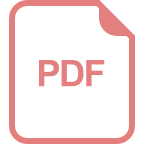
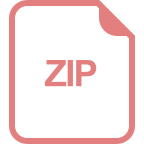
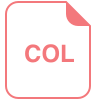
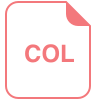






