c++基于opencv的Laplace进行边缘检测
时间: 2023-09-02 18:10:07 浏览: 178
C++基于OpenCV的Laplace边缘检测实现与Python类似,也是使用Laplacian函数进行实现。代码示例如下:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 读取图像并转换为灰度图像
Mat img = imread("image.jpg");
Mat gray;
cvtColor(img, gray, COLOR_BGR2GRAY);
// 计算图像的二阶导数
Mat dst;
Laplacian(gray, dst, CV_16S, 3);
// 对输出图像进行归一化处理
Mat abs_dst;
convertScaleAbs(dst, abs_dst);
// 显示输出图像
imshow("Laplacian", abs_dst);
waitKey(0);
destroyAllWindows();
return 0;
}
```
在C++中,OpenCV的Laplacian函数的参数与Python略有不同。其中,CV_16S表示输出图像的数据类型为16位有符号整数,3表示滤波器的大小。其他部分与Python的代码基本相同。
相关问题
opencv c++边缘检测
以下是两种使用OpenCV C++进行边缘检测的例子:
1. 使用Canny边缘检测算法:
```cpp
#include <opencv2/opencv.hpp>
#include <opencv2/imgproc/imgproc.hpp>
using namespace cv;
int main() {
Mat srcImage = imread("fg.jpg");
imshow("[原图]Canny边缘检测", srcImage);
Mat dstImage, edge, grayImage;
dstImage.create(srcImage.size(), srcImage.type());
cvtColor(srcImage, grayImage, COLOR_BGR2GRAY);
blur(grayImage, edge, Size(3, 3));
Canny(edge, edge, 3, 9, 3);
imshow("[效果图]Canny边缘检测", edge);
waitKey(0);
return 0;
}
```
2. 使用Laplace变换进行边缘检测:
```cpp
#include <opencv2/opencv.hpp>
#include <opencv2/highgui/highgui.hpp>
#include <opencv2/imgproc/imgproc.hpp>
using namespace cv;
int main() {
Mat src, src_gray, dst, abs_dst;
src = imread("fg.jpg");
imshow("【原始图】图像Laplace变换", src);
GaussianBlur(src, src, Size(3, 3), 0, 0, BORDER_DEFAULT);
cvtColor(src, src_gray, CV_RGB2GRAY);
Laplacian(src_gray, dst, CV_16S, 3, 1, 0, BORDER_DEFAULT);
convertScaleAbs(dst, abs_dst);
imshow("【效果图】图像Laplace变换", abs_dst);
waitKey(0);
return 0;
}
```
针对输入的灰度图像,分别采用sobel、laplace或其他算法对图像进行边缘检测。(要求用C或C++语言实现)
以下是C++语言实现Sobel算子和Laplace算子进行边缘检测的示例代码:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 读取图像
Mat image = imread("lena.jpg", IMREAD_GRAYSCALE);
// 判断图像是否读取成功
if (image.empty())
{
cout << "Could not read the image!" << endl;
return -1;
}
// Sobel算子进行边缘检测
Mat sobel_x, sobel_y, sobel;
Sobel(image, sobel_x, CV_16S, 1, 0);
Sobel(image, sobel_y, CV_16S, 0, 1);
convertScaleAbs(sobel_x, sobel_x);
convertScaleAbs(sobel_y, sobel_y);
addWeighted(sobel_x, 0.5, sobel_y, 0.5, 0, sobel);
// Laplace算子进行边缘检测
Mat laplace;
Laplacian(image, laplace, CV_16S);
convertScaleAbs(laplace, laplace);
// 显示结果
imshow("Original Image", image);
imshow("Sobel Edge Detection", sobel);
imshow("Laplace Edge Detection", laplace);
waitKey(0);
return 0;
}
```
在该示例代码中,我们使用了OpenCV库中的Sobel()和Laplacian()函数来实现Sobel算子和Laplace算子进行边缘检测。其中,Sobel()函数用于计算图像的水平和垂直梯度,进而得到梯度幅值和方向,从而实现边缘检测;而Laplacian()函数则是直接计算图像的二阶导数,也可用于边缘检测。
注意:在实际使用中,我们需要根据具体的应用场景和要求选择合适的边缘检测算法,并进行参数调整和优化。
阅读全文
相关推荐
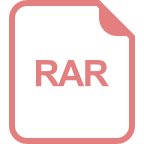
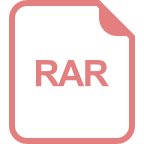
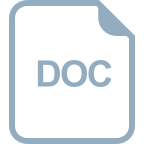
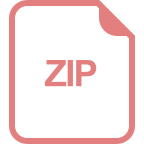
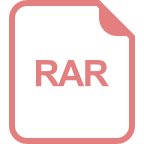
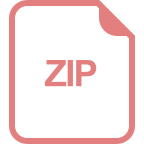
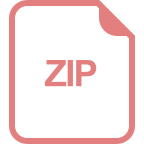
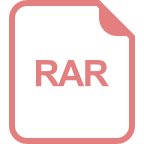
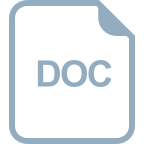
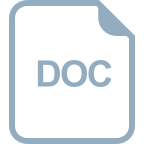
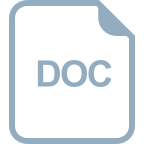
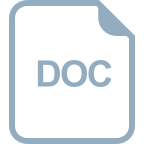
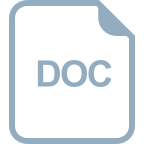
