C++实现Diffie-Hellman算法
时间: 2023-06-19 08:07:41 浏览: 109
Diffie-Hellman算法是一种公钥加密算法,用于安全地交换密钥。下面是C++实现代码:
```c++
#include <iostream>
#include <cmath>
#include <cstdlib>
#include <ctime>
using namespace std;
int gcd(int a, int b)
{
if (b == 0)
return a;
return gcd(b, a % b);
}
int power(int x, unsigned int y, int p)
{
int res = 1;
x = x % p;
while (y > 0) {
if (y & 1)
res = (res*x) % p;
y = y>>1;
x = (x*x) % p;
}
return res;
}
int generate_key(int p)
{
srand((unsigned) time(NULL));
return rand() % (p-2) + 1;
}
int main()
{
int p = 23;
int g = 5;
int a = generate_key(p);
int b = generate_key(p);
cout << "p = " << p << ", g = " << g << endl;
cout << "a = " << a << ", b = " << b << endl;
int A = power(g, a, p);
int B = power(g, b, p);
cout << "A = " << A << ", B = " << B << endl;
int s1 = power(B, a, p);
int s2 = power(A, b, p);
if (s1 == s2)
cout << "Key exchange successful!" << endl;
else
cout << "Key exchange failed!" << endl;
return 0;
}
```
代码中,`gcd()`函数用于求两个数的最大公因数,`power()`函数用于求幂,`generate_key()`函数用于生成随机数。在主函数中,定义了质数`p`和底数`g`,并分别生成了两个随机数`a`和`b`。然后计算出`A`和`B`,并分别计算出两个密钥`s1`和`s2`,最后比较这两个密钥是否相等,判断密钥交换是否成功。
需要注意的是,这里的质数`p`和底数`g`需要事先协商好,而且`p-1`不能是`a`或`b`的因数,否则容易被攻击者破解密钥。
阅读全文
相关推荐
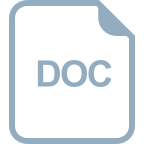
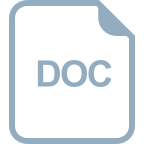
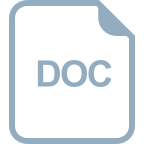



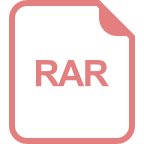
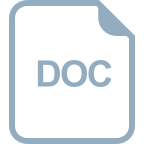
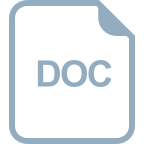


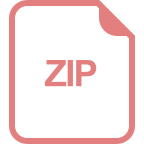
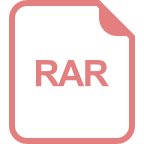
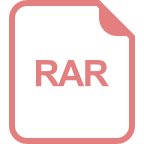
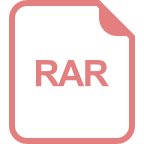
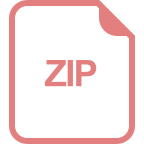
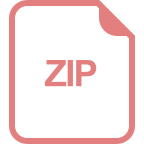
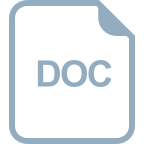
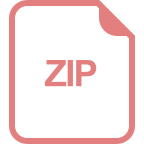