x25519 c++ openssl
时间: 2024-02-02 14:01:35 浏览: 32
x25519是一种用于密钥交换的椭圆曲线加密算法,而OpenSSL是一种开放源代码的密码学库。
x25519是基于曲线25519的Diffie-Hellman密钥交换协议的一种实现。它使用椭圆曲线上的点进行计算,以便双方在不共享实际密钥的情况下生成一个共享的安全密钥。与传统的RSA算法相比,x25519具有更高的计算效率和更小的密钥大小。
而OpenSSL是一个广泛应用于网络安全的软件库,提供了密码学函数、加密算法、SSL/TLS协议实现等功能。它支持许多密码学算法,包括x25519。
在OpenSSL中,可以使用openssl命令行工具生成和管理x25519密钥。例如,可以使用以下命令生成x25519私钥:
openssl genpkey -algorithm x25519 -out private.key
然后,可以使用以下命令从私钥中提取公钥:
openssl pkey -in private.key -pubout -out public.key
生成的公钥可以与其他人共享,以便进行密钥交换。双方可以使用各自的私钥和对方的公钥执行x25519密钥交换。通过计算,双方可以生成一个共享的密钥,用于进行对称加密等操作。
总之,x25519是一种用于密钥交换的椭圆曲线加密算法,而OpenSSL是一个功能强大的密码学库,支持x25519算法以及其他密码学算法的实现。
相关问题
c++ openssl生成证书
在 C++ 中使用 OpenSSL 生成证书可以分为以下几个步骤:
1. 初始化 OpenSSL 库:
```c++
#include <openssl/ssl.h>
#include <openssl/err.h>
// 初始化 OpenSSL 库
SSL_library_init();
SSL_load_error_strings();
OpenSSL_add_all_algorithms();
```
2. 生成证书请求:
```c++
// 创建 X509_REQ 对象
X509_REQ* req = X509_REQ_new();
// 添加公钥到证书请求
EVP_PKEY* pkey = EVP_PKEY_new();
RSA* rsa = RSA_generate_key(2048, RSA_F4, NULL, NULL);
EVP_PKEY_assign_RSA(pkey, rsa);
X509_REQ_set_pubkey(req, pkey);
// 添加主题信息
X509_NAME* name = X509_NAME_new();
X509_NAME_add_entry_by_NID(name, NID_commonName, MBSTRING_UTF8, (unsigned char*)"example.com", -1, -1, 0);
X509_REQ_set_subject_name(req, name);
// 生成证书请求
X509_REQ_sign(req, pkey, EVP_sha256());
```
3. 生成自签名证书:
```c++
// 创建 X509 对象
X509* cert = X509_new();
// 设置证书版本和序列号
ASN1_INTEGER_set(X509_get_serialNumber(cert), 1);
X509_gmtime_adj(X509_get_notBefore(cert), 0);
X509_gmtime_adj(X509_get_notAfter(cert), 31536000L);
// 设置证书请求信息
X509_set_subject_name(cert, X509_REQ_get_subject_name(req));
X509_set_issuer_name(cert, X509_REQ_get_subject_name(req));
X509_set_pubkey(cert, X509_REQ_get_pubkey(req));
// 签名证书
X509_sign(cert, pkey, EVP_sha256());
```
4. 保存证书和私钥:
```c++
// 保存证书
FILE* fp = fopen("cert.pem", "w");
PEM_write_X509(fp, cert);
fclose(fp);
// 保存私钥
fp = fopen("key.pem", "w");
PEM_write_PrivateKey(fp, pkey, NULL, NULL, 0, NULL, NULL);
fclose(fp);
```
注意:在实际使用中,应该对私钥文件进行保护,例如设置只有 root 用户可读写。
windows c++ 引入openssl
在 Windows 上使用 C++ 引入 OpenSSL 可以按照以下步骤进行:
1. 下载 OpenSSL 库
在 OpenSSL 官网的下载页面(https://www.openssl.org/source/)上,选择一个合适的版本下载,推荐下载最新的稳定版本(例如 OpenSSL 1.1.1)。
2. 安装 OpenSSL 库
将下载的 OpenSSL 压缩包解压到某个目录下,例如 `C:\OpenSSL`。
3. 配置 Visual Studio
打开 Visual Studio,创建一个新的 C++ 项目。在项目属性中进行如下配置:
* 将 `C/C++` -> `常规` -> `附加包含目录` 添加 `C:\OpenSSL\include`。
* 将 `链接器` -> `常规` -> `附加库目录` 添加 `C:\OpenSSL\lib`。
* 将 `链接器` -> `输入` -> `附加依赖项` 添加 `libssl.lib;libcrypto.lib`。
4. 使用 OpenSSL 库
在 C++ 代码中引入 OpenSSL 库的头文件,并使用 OpenSSL 库的函数进行编程,例如:
```c++
#include <openssl/bio.h>
#include <openssl/evp.h>
#include <openssl/buffer.h>
// 在这里使用 OpenSSL 库的函数
```
在编译时,需要将 OpenSSL 库的 DLL 文件(`libssl-1_1-x64.dll` 和 `libcrypto-1_1-x64.dll`)拷贝到生成的可执行文件所在的目录下。在 Visual Studio 中,可以将这两个 DLL 文件添加到项目中,并设置为“始终复制”属性,以确保它们被正确地复制到输出目录中。
注意:如果您使用的是静态链接库(.lib 文件),则不需要将 DLL 文件拷贝到输出目录中。
希望这些步骤可以帮助您在 Windows 上使用 C++ 引入 OpenSSL 库。
相关推荐
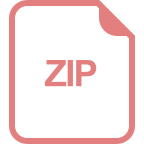
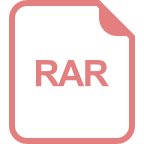












