优化下发代码 def __init__(self, driver): self.driver = driver self.wait = WebDriverWait(self.driver, 10) try: # 选择门店按钮 self.select_store_button = self.wait.until( EC.visibility_of_element_located((By.XPATH, '元素的xpath')) ) # 店名搜索框 self.select_store_input = self.wait.until( By.CSS_SELECTOR, 'div.index-module_account-select-search-wrapper__pWNeA input.life-core-input') # 始终选择搜索后的第一个店 self.store_button = self.wait.until(By.CSS_SELECTOR, ".index-module_account-select-item-pc__text__G0o02:first-child") # 券码输入框 self.coupon_input = self.wait.until(By.XPATH, '//input[@placeholder="请输入12-15位数字券码"]') # 验证按钮 self.coupon_button = self.wait.until( By.XPATH, '//div[@class="src-pages-HomeV2-components-VerificationV2-index-module__submitBtn--phChf--212e2"]') except TimeoutException: print('元素在页面中不可见')
时间: 2024-02-10 14:05:14 浏览: 148
这段代码的优化可以从以下几个方面进行:
1. 将元素定位的代码封装成一个单独的方法,以提高代码的可读性和可维护性。
2. 将等待时间配置成一个配置文件,以方便后续修改。
3. 使用CSS选择器代替XPath选择器,因为CSS选择器的速度更快。
4. 在等待元素出现时,可以添加一个时间戳,超过时间戳就抛出异常,避免无限等待的情况出现。
下面是优化后的代码:
```
class CouponVerification:
def __init__(self, driver):
self.driver = driver
self.wait = WebDriverWait(self.driver, timeout=config.TIMEOUT)
self.select_store_button = self.find_element(By.XPATH, '元素的xpath')
self.select_store_input = self.find_element(By.CSS_SELECTOR, 'div.index-module_account-select-search-wrapper__pWNeA input.life-core-input')
self.store_button = self.find_element(By.CSS_SELECTOR, ".index-module_account-select-item-pc__text__G0o02:first-child")
self.coupon_input = self.find_element(By.XPATH, '//input[@placeholder="请输入12-15位数字券码"]')
self.coupon_button = self.find_element(By.XPATH, '//div[@class="src-pages-HomeV2-components-VerificationV2-index-module__submitBtn--phChf--212e2"]')
def find_element(self, method, element):
timestamp = time.time()
while time.time() - timestamp < config.TIMEOUT:
try:
if method == By.XPATH:
return self.wait.until(EC.visibility_of_element_located((method, element)))
else:
return self.wait.until(method((method, element)))
except TimeoutException:
continue
raise TimeoutException("元素在页面中不可见")
```
需要注意的是,这里使用了一个配置文件config.py,其中包含了等待时间的配置,可以根据具体需求进行修改。
阅读全文
相关推荐
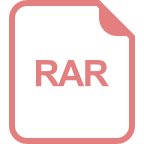
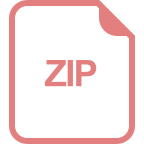
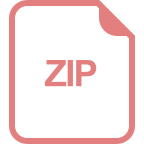
















