请分析以下每一条代码的作用def getHTMLtext(url): """请求获得网页内容""" try: r = requests.get(url, timeout=30) r.raise_for_status() r.encoding = r.apparent_encoding # 是中文正常显示 print("访问成功") return r.text except: print("访问失败") return " "
时间: 2023-06-24 19:08:16 浏览: 128
这段代码定义了一个名为`getHTMLtext`的函数,其功能是通过传入一个url参数获取网页内容。具体分析如下:
- `r = requests.get(url, timeout=30)`:使用requests库的get方法获取指定url的内容,并将结果存储在变量r中。timeout参数设置了请求的超时时间为30秒。
- `r.raise_for_status()`:判断请求是否成功。如果请求返回的状态码为不是200,那么将会引发HTTPError,程序会停止运行。
- `r.encoding = r.apparent_encoding`:使用网页内容的编码方式对r对象进行编码。
- `print("访问成功")`:若请求成功,则输出“访问成功”的提示信息。
- `return r.text`:将网页的文本内容返回。
- `except:`:如果try代码块中的代码出现异常,则执行except代码块中的代码。
- `print("访问失败")`:若请求失败,则输出“访问失败”的提示信息。
- `return " "`:返回一个空字符串。
相关问题
帮我把一下代码设置一个合理请求头,并加入一个延时import requests import os from bs4 import BeautifulSoup class NovelDownloader: def __init__(self, root_url): self.root_url = root_url self.book_list = [] self.chapter_list = [] def get_url(self, url): while True: try: res = requests.get(url) if res.status_code == 200: print("页面获取成功!") return res.text else: print("页面返回异常!", res.status_code) except: print("页面获取错误!") def get_book_list(self): res = self.get_url(self.root_url) html = BeautifulSoup(res, "html.parser") a_list = html.find_all("a", {"class": "name"}) for a in a_list: self.book_list.append(a["href"]) self.book_list = [self.root_url + i for i in self.book_list] self.book_list.remove('http://www.biquge5200.cc/') def get_chapter_list(self, url): res = self.get_url(url) html = BeautifulSoup(res, "html.parser") a_list = html.find_all("a", {"class": "chapter"}) for a in a_list: self.chapter_list.append((a["href"], a.text.replace("\n", ""))) def get_content(self, chapter): url = self.root_url + chapter[0] print(url) book_name = chapter[0].split("/")[1] print(book_name) if not os.path.exists(book_name): os.mkdir(book_name) res = self.get_url(url) html = BeautifulSoup(res, "html.parser") content = html.find("div", {"id": "content"}).text print(content) path = os.path.join(book_name, chapter[1]) with open(path, "w", encoding="utf8") as f: f.write(content) def main(self): self.get_book_list() for book in self.book_list: self.get_chapter_list(book) for chapter in self.chapter_list: self.get_content(chapter) if __name__ == '__main__': root_url = "http://www.biquge5200.cc/" nd = NovelDownloader(root_url) nd.main()
好的,我可以帮你添加一个合理的请求头和延时。在 `get_url` 方法中,你可以添加一个请求头字典,来模拟浏览器发送请求。同时,在每次请求后添加一个时间延时,避免频繁请求被网站屏蔽。
修改后的代码如下所示:
```python
import requests
import os
import time
from bs4 import BeautifulSoup
class NovelDownloader:
def __init__(self, root_url):
self.root_url = root_url
self.book_list = []
self.chapter_list = []
self.headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"
} # 添加请求头
def get_url(self, url):
while True:
try:
res = requests.get(url, headers=self.headers)
if res.status_code == 200:
print("页面获取成功!")
return res.text
else:
print("页面返回异常!", res.status_code)
except:
print("页面获取错误!")
time.sleep(1) # 添加延时
def get_book_list(self):
res = self.get_url(self.root_url)
html = BeautifulSoup(res, "html.parser")
a_list = html.find_all("a", {"class": "name"})
for a in a_list:
self.book_list.append(a["href"])
self.book_list = [self.root_url + i for i in self.book_list]
self.book_list.remove('http://www.biquge5200.cc/')
def get_chapter_list(self, url):
res = self.get_url(url)
html = BeautifulSoup(res, "html.parser")
a_list = html.find_all("a", {"class": "chapter"})
for a in a_list:
self.chapter_list.append((a["href"], a.text.replace("\n", "")))
def get_content(self, chapter):
url = self.root_url + chapter[0]
print(url)
book_name = chapter[0].split("/")[1]
print(book_name)
if not os.path.exists(book_name):
os.mkdir(book_name)
res = self.get_url(url)
html = BeautifulSoup(res, "html.parser")
content = html.find("div", {"id": "content"}).text
print(content)
path = os.path.join(book_name, chapter[1])
with open(path, "w", encoding="utf8") as f:
f.write(content)
def main(self):
self.get_book_list()
for book in self.book_list:
self.get_chapter_list(book)
for chapter in self.chapter_list:
self.get_content(chapter)
if __name__ == '__main__':
root_url = "http://www.biquge5200.cc/"
nd = NovelDownloader(root_url)
nd.main()
```
这样就可以更好地模拟浏览器发送请求,避免被网站屏蔽。另外,可以根据需要调整延时时间,以达到更好的效果。
import requests from bs4 import BeautifulSoup allUniv = [] def getHTMLText(url): try: r = requests.get(url, timeout=30) r.raise_for_status() r.encoding = 'utf-8' return r.text except: return "" def fillUnivList(soup): data = soup.find_all('tr') for tr in data: ltd = tr.find_all('td') if len(ltd)==0: continue singleUniv = [] for td in ltd: singleUniv.append(td.string) allUniv.append(singleUniv) def printUnivList(num): print("{:^4}{:^10}{:^5}{:^8}{:^10}".format("排名","学校名称","省市","总分","培养规模")) for i in range(num): u=allUniv[i] print("{:^4}{:^10}{:^5}{:^8}{:^10}".format(u[0],u[1],u[2],u[3],u[6])) def main(): url = 'http://www.zuihaodaxue.cn/zuihaodaxuepaiming2016.html' html = getHTMLText(url) soup = BeautifulSoup(html, "html.parser") fillUnivList(soup) printUnivList(10) main()
这段代码是用来爬取2016年全国大学排名前10的大学的信息,并输出到控制台上。代码首先定义了一个空列表 allUniv,然后定义了三个函数:getHTMLText、fillUnivList、printUnivList。其中,getHTMLText函数用来获取指定URL的HTML文本内容,fillUnivList函数用来解析HTML文本内容并将排名前10大学的信息保存到 allUniv 列表中,printUnivList函数用来将 allUniv 列表中的信息输出到控制台上。最后,main函数调用了以上三个函数,从而完成了爬取和输出的任务。
阅读全文
相关推荐
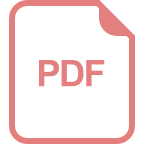
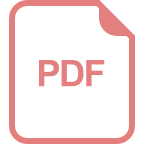














