能将这个代码帮我注释一下吗?import tkinter as tk import requests def scan(): url = entry.get() if not url.startswith("http"): url = "http://" + url try: response = requests.get(url) if response.status_code == 200: result.config(text="目录扫描成功!") else: result.config(text="目录扫描失败!") except: result.config(text="目录扫描失败!") root = tk.Tk() root.title("Web目录扫描器") label = tk.Label(root, text="请输入URL:") label.pack() entry = tk.Entry(root, width=50) entry.pack() button = tk.Button(root, text="扫描", command=scan) button.pack() result = tk.Label(root, text="") result.pack() root.mainloop()
时间: 2023-05-16 13:06:41 浏览: 73
这是一个使用 tkinter 和 requests 库编写的 Web 目录扫描器,它可以扫描指定 URL 的目录,并返回扫描结果。在程序中,用户需要输入要扫描的 URL,然后点击“扫描”按钮即可开始扫描。如果扫描成功,程序会显示“目录扫描成功!”的提示信息,否则会显示“目录扫描失败!”的提示信息。如果您需要注释这个代码,可以在每个函数和语句的上方添加注释,以便更好地理解代码的功能和作用。
相关问题
import tkinter as tk import requests def scan(): url = entry.get() if not url.startswith("http"): url = "http://" + url try: response = requests.get(url) if response.status_code == 200: result.config(text="目录扫描成功!") else: result.config(text="目录扫描失败!") except: result.config(text="目录扫描失败!") root = tk.Tk() root.title("Web目录扫描器") label = tk.Label(root, text="请输入URL:") label.pack() entry = tk.Entry(root, width=50) entry.pack() button = tk.Button(root, text="扫描", command=scan) button.pack() result = tk.Label(root, text="") result.pack() root.mainloop() 帮我写个注释
# 导入tkinter和requests模块
import tkinter as tk
import requests
# 定义一个函数,用于扫描目录
def scan():
# 获取用户输入的URL
url = entry.get()
# 如果URL不以"http"开头,则添加"http://"前缀
if not url.startswith("http"):
url = "http://" + url
try:
# 发送HTTP请求,获取响应
response = requests.get(url)
# 如果响应状态码为200,则表示目录扫描成功
if response.status_code == 200:
result.config(text="目录扫描成功!")
# 否则表示目录扫描失败
else:
result.config(text="目录扫描失败!")
# 如果发生异常,则表示目录扫描失败
except:
result.config(text="目录扫描失败!")
# 创建一个窗口
root = tk.Tk()
# 设置窗口标题
root.title("Web目录扫描器")
# 创建一个标签,用于提示用户输入URL
label = tk.Label(root, text="请输入URL:")
label.pack()
# 创建一个文本框,用于接收用户输入的URL
entry = tk.Entry(root, width=50)
entry.pack()
# 创建一个按钮,用于触发目录扫描
button = tk.Button(root, text="扫描", command=scan)
button.pack()
# 创建一个标签,用于显示目录扫描结果
result = tk.Label(root, text="")
result.pack()
# 进入消息循环,等待用户操作
root.mainloop()
import tkinter as tk import requests from bs4 import BeautifulSoup def scan(): url = entry.get() res = requests.get(url) soup = BeautifulSoup(res.text, 'html.parser') links = soup.find_all('a') for link in links: print(link.get('href')) root = tk.Tk() root.title('Web Scanner') label = tk.Label(root, text='Enter URL:') label.pack() entry = tk.Entry(root) entry.pack() button = tk.Button(root, text='Scan', command=scan) button.pack() root.mainloop() 帮我写一个注释
# 导入必要的模块
import tkinter as tk
import requests
from bs4 import BeautifulSoup
# 定义一个函数,用于扫描网页并输出链接
def scan():
# 获取用户输入的网址
url = entry.get()
# 发送请求并获取响应内容
res = requests.get(url)
# 使用 BeautifulSoup 解析响应内容
soup = BeautifulSoup(res.text, 'html.parser')
# 查找所有的链接标签
links = soup.find_all('a')
# 遍历所有的链接标签并输出链接
for link in links:
print(link.get('href'))
# 创建一个窗口对象
root = tk.Tk()
# 设置窗口标题
root.title('Web Scanner')
# 创建一个标签对象并添加到窗口中
label = tk.Label(root, text='Enter URL:')
label.pack()
# 创建一个文本框对象并添加到窗口中
entry = tk.Entry(root)
entry.pack()
# 创建一个按钮对象并添加到窗口中
button = tk.Button(root, text='Scan', command=scan)
button.pack()
# 进入消息循环,等待用户操作
root.mainloop()
阅读全文
相关推荐
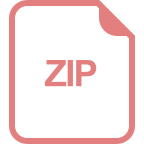
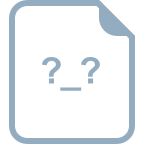
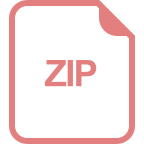







帮我增加进度条import io import re import tkinter import requests import threading from pydub import AudioSegment root = tkinter.Tk() root.title('在线视频解析') root.geometry('500x590+550+350') headers = { 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:109.0) Gecko/20100101 Firefox/115.0'} ac = tkinter.Listbox(root, width=50, height=20, font=('黑体', 12)) ac.grid(row=2, columnspan=10, sticky="n" + "s" + "w" + "e") def sousuo(): i = b1.get() ac.delete(0, 'end') def extract_music_info(content): p = '|' content = re.sub(p, '', content, flags=re.S) pattern = re.compile('subject.*?href="(.*?)">(.*?)
', flags=re.S) return pattern.findall(content) def search_music(): url = 'https://www.hifini.com/search-' + i + '-1.htm' response = requests.get(url=url, headers=headers) return response.text def update_listbox(music_list): for music in music_list: pppp = music[1] + ":" + music[0] ac.insert('end', pppp) content = search_music() music_list = extract_music_info(content) update_listbox(music_list) def xiazzi(): def download_music(): ppp = ac.get(ac.curselection()) pp = re.search('thread.*?htm', ppp) v = pp.group() url1 = 'https://www.hifini.com/' + v response = requests.get(url=url1, headers=headers) ppp = response.text l2 = re.search('<script>.*?title:..(.*?).,.*?author:.(.*?).,.*?url:..(.*?).,', ppp, flags=re.S) p = 'https://www.hifini.com/' + l2.group(3) response = requests.get(url=p, headers=headers) l3 = response.content music_name = '{}-{}.mp3'.format(l2.group(2), l2.group(1)) if l3.startswith(b'\x00\x00\x00\x20\x66\x74\x79\x70'): audio = AudioSegment.from_file(io.BytesIO(l3), format='m4a') audio.export(music_name, format='mp3') else: with open(music_name, 'wb') as f: f.write(l3) print(music_name) threading.Thread(target=download_music).start() a1 = tkinter.Label(root, text='音乐下载器', anchor="center", font=('黑体', 24)) a1.grid(row=0, columnspan=10, sticky="n" + "s" + "w" + "e") b1 = tkinter.Entry(root, width=35, font=('黑体', 16), ) b1.grid(row=1, column=3, padx=15) search_button = tkinter.Button(root, text='搜索', command=sousuo) search_button.grid(row=1, column=4) download_button = tkinter.Button(root, text='下载', command=xiazzi) download_button.grid(row=3, column=4) root.mainloop()
import io import re import tkinter import requests import threading import tqdm from pydub import AudioSegment root = tkinter.Tk() root.title('在线视频解析') root.geometry('500x590+550+350') headers = { 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:109.0) Gecko/20100101 Firefox/115.0'} ac = tkinter.Listbox(root, width=50, height=20, font=('黑体', 12)) ac.grid(row=2, columnspan=10, sticky="n" + "s" + "w" + "e") def sousuo(): i = b1.get() ac.delete(0, 'end') def extract_music_info(content): p = '|' content = re.sub(p, '', content, flags=re.S) pattern = re.compile('subject.*?href="(.*?)">(.*?)
', flags=re.S) return pattern.findall(content) def search_music(): url = 'https://www.hifini.com/search-' + i + '-1.htm' response = requests.get(url=url, headers=headers) return response.text def update_listbox(music_list): for music in music_list: pppp = music[1] + ":" + music[0] ac.insert('end', pppp) content = search_music() music_list = extract_music_info(content) update_listbox(music_list) def xiazzi(): def download_music(): ppp = ac.get(ac.curselection()) pp = re.search('thread.*?htm', ppp) v = pp.group() url1 = 'https://www.hifini.com/' + v response = requests.get(url=url1, headers=headers) ppp = response.text l2 = re.search('<script>.*?title:..(.*?).,.*?author:.(.*?).,.*?url:..(.*?).,', ppp, flags=re.S) p = 'https://www.hifini.com/' + l2.group(3) response = requests.get(url=p, headers=headers, stream=True) # 设置 stream=True 以启用流式下载 total_size = int(response.headers.get('Content-Length')) music_name = '{}-{}.mp3'.format(l2.group(2), l2.group(1)) progress_bar = tqdm(total=total_size, unit='B', unit_scale=True) # 创建进度条 with open(music_name, 'wb') as f: for data in response.iter_content(chunk_size=1024): progress_bar.update(len(data)) # 更新进度条 f.write(data) progress_bar.close() # 关闭进度条 print(music_name) threading.Thread(target=download_music).start() a1 = tkinter.Label(root, text='音乐下载器', anchor="center", font=('黑体', 24)) a1.grid(row=0, columnspan=10, sticky="n" + "s" + "w" + "e") b1 = tkinter.Entry(root, width=35, font=('黑体', 16), ) b1.grid(row=1, column=3, padx=15) search_button = tkinter.Button(root, text='搜索', command=sousuo) search_button.grid(row=1, column=4) download_button = tkinter.Button(root, text='下载', command=xiazzi) download_button.grid(row=3, column=4) root.mainloop() 报错TypeError: 'module' object is not callable
import re,tkinter,requests,threading,tqdm as tt root = tkinter.Tk() root.title('在线视频解析') root.geometry('500x590+550+350') headers = { 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:109.0) Gecko/20100101 Firefox/115.0'} ac = tkinter.Listbox(root, width=50, height=20, font=('黑体', 12)) ac.grid(row=2, columnspan=10, sticky="n" + "s" + "w" + "e") def sousuo(): i = b1.get() ac.delete(0, 'end') def extract_music_info(content): p = '|' content = re.sub(p, '', content, flags=re.S) pattern = re.compile('subject.*?href="(.*?)">(.*?)
', flags=re.S) return pattern.findall(content) def search_music(): url = 'https://www.hifini.com/search-' + i + '-1.htm' response = requests.get(url=url, headers=headers) return response.text def update_listbox(music_list): for music in music_list: pppp = music[1] + ":" + music[0] ac.insert('end', pppp) content = search_music() music_list = extract_music_info(content) update_listbox(music_list) def xiazzi(): def download_music(): ppp = ac.get(ac.curselection()) pp = re.search('thread.*?htm', ppp) v = pp.group() url1 = 'https://www.hifini.com/' + v response = requests.get(url=url1, headers=headers) ppp = response.text l2 = re.search('<script>.*?title:..(.*?).,.*?author:.(.*?).,.*?url:..(.*?).,', ppp, flags=re.S) p = 'https://www.hifini.com/' + l2.group(3) response = requests.get(url=p, headers=headers, stream=True) # 设置 stream=True 以启用流式下载 total_size = int(response.headers.get('Content-Length')) music_name = '{}-{}.mp3'.format(l2.group(2), l2.group(1)) progress_bar = tt.tqdm(total=total_size, unit='B', unit_scale=True) # 创建进度条 with open(music_name, 'wb') as f: for data in response.iter_content(chunk_size=1024): progress_bar.update(len(data)) # 更新进度条 f.write(data) progress_bar.close() # 关闭进度条 print(music_name) threading.Thread(target=download_music).start() a1 = tkinter.Label(root, text='音乐下载器', anchor="center", font=('黑体', 24)) a1.grid(row=0, columnspan=10, sticky="n" + "s" + "w" + "e") b1 = tkinter.Entry(root, width=35, font=('黑体', 16), ) b1.grid(row=1, column=3, padx=15) search_button = tkinter.Button(root, text='搜索', command=sousuo) search_button.grid(row=1, column=4) download_button = tkinter.Button(root, text='下载', command=xiazzi) download_button.grid(row=3, column=4) root.mainloop() 将download_button带有下载行为的按钮添加进列表,




