java netty nio
时间: 2023-10-04 11:13:26 浏览: 67
Java BIO是一种传统的Java IO编程方式,采用同步阻塞的方式进行数据传输。在BIO模式下,服务器为每个连接启动一个线程进行处理,如果连接处于空闲状态,会造成不必要的线程开销。BIO适用于连接数较小且固定的架构,对服务器资源要求较高,且并发性受限。在JDK1.4之前,BIO是唯一的选择,因为其编程简单易理解。
Netty是一个开源的Java网络通信框架,它提供了异步非阻塞的网络编程模型。与BIO不同,Netty采用NIO模型进行实现,可以高效地处理大量的并发连接。Netty提供了更高级的抽象和功能,使开发人员能够更轻松地构建可扩展和高性能的网络应用程序。
相关问题
用java netty nio 写一个tcpserver
好的,以下是一个使用Java Netty NIO编写的TCP服务器的基本示例代码:
```java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
public class TCPServer {
private final int port;
public TCPServer(int port) {
this.port = port;
}
public void run() throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new TCPServerHandler());
}
});
ChannelFuture f = b.bind(port).sync();
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
int port = Integer.parseInt(args[0]);
new TCPServer(port).run();
}
}
```
在这个示例中,我们使用了Java Netty框架,它提供了一种快速构建网络应用程序的方式。我们创建了一个名为“TCPServer”的类,它包含一个构造函数和三个方法:run(),main()和一个自定义的“TCPServerHandler”类。
在“run()”方法中,我们创建了两个事件循环组:bossGroup和workerGroup。bossGroup接受来自客户端的连接,workerGroup负责处理实际的I/O操作。然后,我们创建了一个ServerBootstrap实例,并将bossGroup和workerGroup分别设置为其组。接下来,我们指定了服务器通道类型(NioServerSocketChannel),并设置了一个ChannelInitializer,该初始化程序将添加一个自定义的TCPServerHandler来处理来自客户端的消息。
在“main()”方法中,我们将创建一个新的TCPServer实例,并调用其“run()”方法。在该方法中,我们绑定服务器到指定的端口,并等待直到服务器通道关闭。
最后,我们有一个自定义的“TCPServerHandler”类,它实现了Netty的ChannelInboundHandler接口。该处理程序将处理来自客户端的消息,并在收到消息时向客户端发送响应。以下是该处理程序类的示例代码:
```java
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInboundHandlerAdapter;
public class TCPServerHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) {
ByteBuf in = (ByteBuf) msg;
try {
while (in.isReadable()) {
System.out.print((char) in.readByte());
System.out.flush();
}
} finally {
in.release();
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
cause.printStackTrace();
ctx.close();
}
}
```
在这个示例中,我们实现了Netty的ChannelInboundHandler接口,并重写了其中的“channelRead()”和“exceptionCaught()”方法。在“channelRead()”方法中,我们接收来自客户端的消息,并将其打印到控制台。在“exceptionCaught()”方法中,我们捕获任何异常并关闭通道。
这就是一个简单的Java Netty NIO TCP服务器的基本示例。
用java netty nio 写一个tcpserver和一个httpserver,tcpservers使用端口8000,httpserver使用端口8900
好的,以下是基于Java Netty NIO框架实现的TCP Server和HTTP Server的代码:
TCP Server:
```java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
public class TCPServer {
private int port;
public TCPServer(int port) {
this.port = port;
}
public void run() throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new TCPServerHandler());
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
ChannelFuture f = b.bind(port).sync();
System.out.println("TCP Server started on port " + port);
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
int port = 8000;
new TCPServer(port).run();
}
}
```
HTTP Server:
```java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
import io.netty.handler.codec.http.HttpObjectAggregator;
import io.netty.handler.codec.http.HttpServerCodec;
import io.netty.handler.codec.http.HttpServerExpectContinueHandler;
public class HTTPServer {
private int port;
public HTTPServer(int port) {
this.port = port;
}
public void run() throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new HttpServerCodec());
ch.pipeline().addLast(new HttpServerExpectContinueHandler());
ch.pipeline().addLast(new HttpObjectAggregator(65536));
ch.pipeline().addLast(new HTTPServerHandler());
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
ChannelFuture f = b.bind(port).sync();
System.out.println("HTTP Server started on port " + port);
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
int port = 8900;
new HTTPServer(port).run();
}
}
```
需要注意的是,以上代码中的 `TCPServerHandler` 和 `HTTPServerHandler` 分别是处理 TCP 和 HTTP 请求的自定义处理器,需要根据实际需求编写。
相关推荐
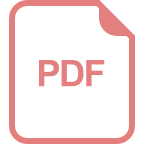












