编写matplotlib 绘制动态折线图程序
时间: 2023-04-01 17:03:44 浏览: 95
好的,我可以回答这个问题。编写matplotlib 绘制动态折线图程序的代码如下:
```
import matplotlib.pyplot as plt
import numpy as np
# 创建一个空的折线图
fig, ax = plt.subplots()
line, = ax.plot([], [], lw=2)
# 设置x轴和y轴的范围
ax.set_xlim(, 10)
ax.set_ylim(-1, 1)
# 生成x轴的数据
x = np.linspace(, 10, 100)
# 定义一个更新函数,用于更新折线图的数据
def update(num):
y = np.sin(x + num / 10.)
line.set_data(x, y)
return line,
# 创建动画
from matplotlib.animation import FuncAnimation
ani = FuncAnimation(fig, update, frames=100, interval=50, blit=True)
# 显示折线图
plt.show()
```
这段代码可以生成一个动态的折线图,其中折线的形状会随着时间的推移而变化。
相关问题
用python编写一个获取数据并绘制实时动态折线图的程序
好的,以下是一个使用Python的matplotlib库和pandas库实现获取数据并实时绘制动态折线图的简单示例代码:
```python
import pandas as pd
import matplotlib.pyplot as plt
# 创建一个空的pandas DataFrame
df = pd.DataFrame(columns=['x', 'y'])
# 创建一个matplotlib折线图
plt.ion()
fig = plt.figure()
ax = fig.add_subplot(111)
line1, = ax.plot(df['x'], df['y'], 'r-')
# 模拟数据源,每隔1秒生成一组随机数据
import random
import time
while True:
x = len(df)
y = random.randint(0, 100)
df.loc[x] = [x, y]
# 更新折线图
line1.set_xdata(df['x'])
line1.set_ydata(df['y'])
ax.relim()
ax.autoscale_view()
fig.canvas.draw()
fig.canvas.flush_events()
# 暂停1秒
time.sleep(1)
```
这个示例程序会每隔1秒生成一组随机数据,并实时绘制折线图。你可以将数据源替换为你自己的数据源,然后运行这个程序即可实现实时动态折线图的绘制。
pyecharts和matplotlib在绘制地图上的区别
Pyecharts 和 Matplotlib 都可以用来绘制地图,但是两者在绘制地图方面还是有一些区别的。
Pyecharts 是一个基于 Echarts.js 的 Python 可视化库,它提供了很多种绘图类型,包括地图、柱状图、折线图等等。对于地图的绘制,Pyecharts 提供了很多地图类型(如中国地图、世界地图等),并且支持地图的数据可视化,例如可以根据不同区域的数据进行颜色填充、气泡大小显示等等。
Matplotlib 是一个 Python 的绘图库,它可以绘制各种类型的图表,包括线图、柱状图、散点图、等高线图等等。对于地图的绘制,Matplotlib 可以通过 Basemap 工具包来实现,可以绘制各种类型的地图,包括等距投影、极地投影、墨卡托投影等等。Matplotlib 的地图绘制相对来说比较灵活,但是需要编写较多的代码。
总的来说,Pyecharts 对于地图数据可视化方面更加友好,而 Matplotlib 对于地图绘制方面更加灵活,但需要编写较多的代码。
相关推荐
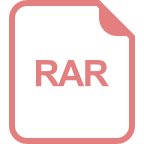
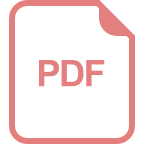
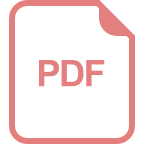












