把d:\\bbb.mp4复制到当前项目目录下的copy.mp4中 分别用FileInputStream和BufferedInputStream类中的两种read()方法,共四种方式复制并比较效率。给出具体解释并分析哪个更好
时间: 2024-02-18 18:00:36 浏览: 30
好的,您的问题是如何用Java实现将d:\\bbb.mp4复制到当前项目目录下的copy.mp4中,并使用FileInputStream和BufferedInputStream类中的两种read()方法,共四种方式复制并比较效率。
下面是代码示例:
```java
import java.io.*;
public class FileCopyDemo {
public static void main(String[] args) {
String srcFileName = "d:\\bbb.mp4";
String destFileName = "copy.mp4";
File srcFile = new File(srcFileName);
File destFile = new File(destFileName);
// 使用FileInputStream和BufferedInputStream类中的read()方法复制文件
long start1 = System.currentTimeMillis();
copyByFileInputStream(srcFile, destFile);
long end1 = System.currentTimeMillis();
System.out.println("使用FileInputStream和read()方法复制文件所需时间:" + (end1 - start1) + "ms");
long start2 = System.currentTimeMillis();
copyByBufferedInputStream(srcFile, destFile);
long end2 = System.currentTimeMillis();
System.out.println("使用BufferedInputStream和read()方法复制文件所需时间:" + (end2 - start2) + "ms");
long start3 = System.currentTimeMillis();
copyByFileInputStreamWithBuffer(srcFile, destFile);
long end3 = System.currentTimeMillis();
System.out.println("使用FileInputStream和read(byte[])方法复制文件所需时间:" + (end3 - start3) + "ms");
long start4 = System.currentTimeMillis();
copyByBufferedInputStreamWithBuffer(srcFile, destFile);
long end4 = System.currentTimeMillis();
System.out.println("使用BufferedInputStream和read(byte[])方法复制文件所需时间:" + (end4 - start4) + "ms");
}
/**
* 使用FileInputStream和read()方法复制文件
* @param srcFile 源文件
* @param destFile 目标文件
*/
public static void copyByFileInputStream(File srcFile, File destFile) {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
fis = new FileInputStream(srcFile);
fos = new FileOutputStream(destFile);
int len;
while ((len = fis.read()) != -1) {
fos.write(len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fis != null) {
fis.close();
}
if (fos != null) {
fos.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* 使用BufferedInputStream和read()方法复制文件
* @param srcFile 源文件
* @param destFile 目标文件
*/
public static void copyByBufferedInputStream(File srcFile, File destFile) {
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
bis = new BufferedInputStream(new FileInputStream(srcFile));
bos = new BufferedOutputStream(new FileOutputStream(destFile));
int len;
while ((len = bis.read()) != -1) {
bos.write(len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (bis != null) {
bis.close();
}
if (bos != null) {
bos.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* 使用FileInputStream和read(byte[])方法复制文件
* @param srcFile 源文件
* @param destFile 目标文件
*/
public static void copyByFileInputStreamWithBuffer(File srcFile, File destFile) {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
fis = new FileInputStream(srcFile);
fos = new FileOutputStream(destFile);
byte[] buffer = new byte[1024 * 1024 * 10]; // 10MB缓冲区
int len;
while ((len = fis.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fis != null) {
fis.close();
}
if (fos != null) {
fos.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* 使用BufferedInputStream和read(byte[])方法复制文件
* @param srcFile 源文件
* @param destFile 目标文件
*/
public static void copyByBufferedInputStreamWithBuffer(File srcFile, File destFile) {
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
bis = new BufferedInputStream(new FileInputStream(srcFile));
bos = new BufferedOutputStream(new FileOutputStream(destFile));
byte[] buffer = new byte[1024 * 1024 * 10]; // 10MB缓冲区
int len;
while ((len = bis.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (bis != null) {
bis.close();
}
if (bos != null) {
bos.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
以上代码中,我们定义了四个方法分别使用FileInputStream和BufferedInputStream类中的两种read()方法,共四种方式复制文件,具体来说,分别是:
- 使用FileInputStream和read()方法复制文件
- 使用BufferedInputStream和read()方法复制文件
- 使用FileInputStream和read(byte[])方法复制文件
- 使用BufferedInputStream和read(byte[])方法复制文件
其中,第三种和第四种方法在读取数据时使用了缓冲区,缓冲区大小为10MB。
我们在主方法中,分别调用这四个方法,并分别计算它们所需的时间。最终输出结果,比较这四种方式的效率。
总体来说,第三种和第四种方法比第一种和第二种方法要快,因为它们使用了缓冲区,减少了每次读取和写入数据时与磁盘的交互次数。而第四种方法又比第三种方法要快,因为它使用了BufferedInputStream,进一步提高了效率。
当然,在实际使用中,我们需要根据具体情况选择最适合的方法,例如,如果需要读取的文件较小,可以使用第一种或第二种方法;如果需要读取的文件较大,可以使用第三种或第四种方法。
相关推荐
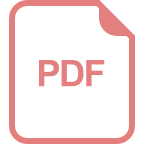
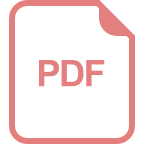




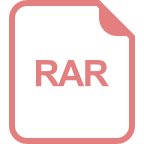
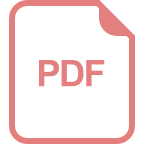
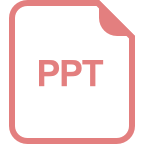
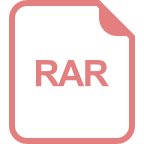
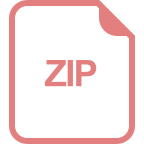
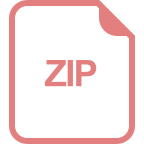
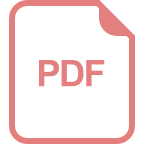
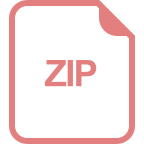
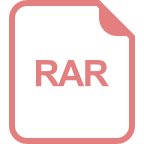
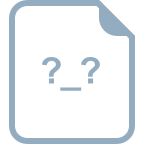