1.基于面向对象思想,分二级建立王者荣耀游戏中的英雄类(抽象的英雄、特定类型的英雄)。 2.在类中对英雄的属性(比如英雄的种类、魔法值、生命值、物理攻击值)及行为(比如魔法攻击、物理攻击)进行具体描述。 3.随机建立两个英雄,设计PK规则,实现两个英雄的PK,直至分开胜负,并且在PK过程中输出两个英雄的状态变化。用c++卸除代码
时间: 2024-03-22 12:43:01 浏览: 16
下面是基于面向对象思想的王者荣耀游戏中英雄类的代码,包括英雄类的属性和行为,以及两个英雄的PK规则和状态变化输出:
```c++
#include <iostream>
#include <string>
#include <cstdlib>
#include <ctime>
using namespace std;
// 英雄类
class Hero {
public:
// 构造函数
Hero(string n, string t) : name(n), type(t), hp(100), mp(50), atk(10) {}
// 获取英雄名称
string getName() {
return name;
}
// 获取英雄类型
string getType() {
return type;
}
// 获取英雄生命值
int getHP() {
return hp;
}
// 获取英雄魔法值
int getMP() {
return mp;
}
// 获取英雄物理攻击力
int getATK() {
return atk;
}
// 物理攻击
void physicalAttack(Hero &target) {
int damage = this->getATK();
target.hp -= damage;
cout << this->getName() << "对" << target.getName() << "造成了" << damage << "点物理伤害。" << endl;
}
// 魔法攻击
void magicAttack(Hero &target) {
int cost = 10;
if (this->mp < cost) {
cout << this->getName() << "法力不足,无法使用魔法攻击!" << endl;
return;
}
int damage = this->getATK() + 10;
target.hp -= damage;
this->mp -= cost;
cout << this->getName() << "对" << target.getName() << "造成了" << damage << "点魔法伤害。" << endl;
}
private:
string name; // 英雄名称
string type; // 英雄类型
int hp; // 生命值
int mp; // 魔法值
int atk; // 物理攻击力
};
int main() {
srand(time(nullptr));
// 随机选择两个英雄
Hero heroes[5] = {
Hero("程咬金", "坦克"),
Hero("貂蝉", "法师"),
Hero("孙尚香", "射手"),
Hero("曹操", "战士"),
Hero("韩信", "刺客")
};
int idx1 = rand() % 5;
int idx2 = rand() % 5;
while (idx1 == idx2) {
idx2 = rand() % 5;
}
Hero &hero1 = heroes[idx1];
Hero &hero2 = heroes[idx2];
cout << "随机选择了两个英雄:" << endl;
cout << hero1.getName() << "(" << hero1.getType() << ")" << endl;
cout << hero2.getName() << "(" << hero2.getType() << ")" << endl;
// PK过程
int rounds = 1;
while (hero1.getHP() > 0 && hero2.getHP() > 0) {
cout << "第" << rounds << "回合:" << endl;
int action1 = rand() % 2;
int action2 = rand() % 2;
if (action1 == 0) {
hero1.physicalAttack(hero2);
} else {
hero1.magicAttack(hero2);
}
if (action2 == 0) {
hero2.physicalAttack(hero1);
} else {
hero2.magicAttack(hero1);
}
cout << hero1.getName() << "的生命值:" << hero1.getHP() << endl;
cout << hero2.getName() << "的生命值:" << hero2.getHP() << endl;
rounds++;
}
// 输出胜者
if (hero1.getHP() > 0) {
cout << hero1.getName() << "获胜!" << endl;
} else {
cout << hero2.getName() << "获胜!" << endl;
}
return 0;
}
```
在上面的代码中,我们随机选择了两个英雄,然后进行了多轮PK,直到一方生命值为0,输出胜者。在PK过程中,我们随机选择物理攻击或魔法攻击,并根据攻击类型和攻击力计算伤害,并输出状态变化。
相关推荐
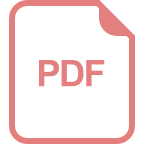
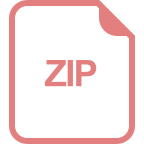















