使用C语言写一个读写串口/dev/ttyS0的程序
时间: 2023-12-13 08:03:08 浏览: 167
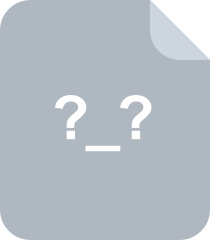
用C语言编写串口程序
好的,以下是一个简单的使用C语言读写/dev/ttyS0串口的程序示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#include <string.h>
int main()
{
int fd; // 串口文件描述符
struct termios options; // 串口设置结构体
char buf[256]; // 读取数据缓存区
// 打开串口设备文件
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("open");
exit(1);
}
// 设置串口属性
tcgetattr(fd, &options);
cfsetispeed(&options, B9600); // 设置输入波特率为9600
cfsetospeed(&options, B9600); // 设置输出波特率为9600
options.c_cflag |= (CLOCAL | CREAD); // 必须设置的标志
options.c_cflag &= ~PARENB; // 无奇偶校验位
options.c_cflag &= ~CSTOPB; // 1位停止位
options.c_cflag &= ~CSIZE; // 字符长度为8位
options.c_cflag |= CS8;
options.c_lflag &= ~(ICANON | ECHO | ECHOE); // 设置为原始模式
options.c_cc[VTIME] = 0; // 非规范模式读取时的超时时间(单位为100毫秒)
options.c_cc[VMIN] = 1; // 非规范模式读取时的最小字符数
tcsetattr(fd, TCSANOW, &options); // 保存设置
// 写入数据
char *data = "hello";
int len = strlen(data);
write(fd, data, len);
// 读取数据
memset(buf, 0, sizeof(buf));
int n = read(fd, buf, sizeof(buf));
if (n < 0) {
perror("read");
exit(1);
} else if (n == 0) {
printf("No data on serial\n");
} else {
printf("Received data: %s\n", buf);
}
// 关闭串口设备文件
close(fd);
return 0;
}
```
该程序首先打开/dev/ttyS0设备文件,并使用tcgetattr()和tcsetattr()函数设置串口属性,然后使用write()函数向串口写入数据,最后使用read()函数从串口读取数据。需要注意的是,在使用read()函数读取数据时,如果串口中没有数据可读,则read()函数将会阻塞,直到有数据可读或出现错误。该程序中使用了非规范模式读取串口数据,即设置了VTIME和VMIN参数,这样read()函数在读取时,如果在VTIME时间内没有读取到VMIN个字符,则会返回0,否则返回读取到的字符数。
阅读全文
相关推荐
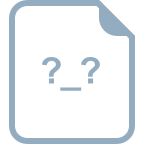





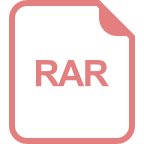
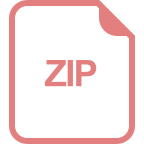
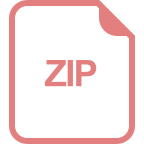
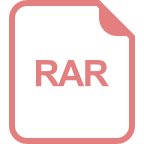
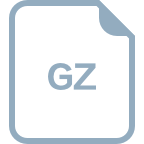
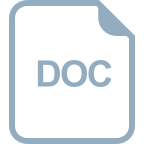



