用C语言写一段通过串口发送数组的代码
时间: 2023-08-31 20:23:46 浏览: 75
### 回答1:
一个可以用于发送数组数据的C语言代码如下:#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>int main()
{
int fd;
int i;
unsigned char array[10] = {0xAA, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09, 0xAA};
struct termios options; fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
tcsetattr(fd, TCSANOW, &options); for(i=0; i<10; i++)
{
write(fd, &array[i], 1);
} close(fd);
return 0;
}
### 回答2:
下面是一个使用C语言编写的通过串口发送数组的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main() {
int fd;
char buffer[16] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16};
// 打开串口
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (fd == -1) {
perror("无法打开串口");
return 1;
}
// 配置串口参数
struct termios serial;
memset(&serial, 0, sizeof(serial));
serial.c_cflag = B9600 | CS8 | CREAD | CLOCAL;
tcsetattr(fd, TCSANOW, &serial);
// 发送数据
write(fd, buffer, sizeof(buffer));
// 关闭串口
close(fd);
return 0;
}
```
以上代码使用了Linux系统的串口设备文件`/dev/ttyS0`,如果你的系统上的串口设备文件命名不同,你需要根据实际情况进行修改。在实际使用时,可以根据需要修改串口参数,如波特率`B9600`等。数组`buffer`中存储了要发送的数据,使用`write`函数将数据发送到串口。之后通过`close`函数关闭串口。请确保以管理员权限运行程序。记得在程序中进行错误处理,以防止出现错误情况。
### 回答3:
下面是一段使用C语言编写的通过串口发送数组的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main()
{
int serial_fd;
char *portname = "/dev/ttyUSB0"; // 根据实际情况选择串口设备地址
int baudrate = B9600; // 波特率设置为9600bps
int data_array[] = {1, 2, 3, 4, 5}; // 要发送的数据数组
int array_length = sizeof(data_array) / sizeof(int); // 数组长度
int bytes_written;
serial_fd = open(portname, O_WRONLY | O_NOCTTY | O_SYNC);
if (serial_fd < 0)
{
perror("无法打开串口");
exit(1);
}
struct termios tty;
memset(&tty, 0, sizeof(tty));
if (tcgetattr(serial_fd, &tty) != 0)
{
perror("无法获取串口属性");
exit(1);
}
cfsetospeed(&tty, baudrate); // 设置波特率
tty.c_cflag |= (CLOCAL | CREAD); // 允许读写
tty.c_cflag &= ~CSIZE;
tty.c_cflag |= CS8; // 数据位为8位
tty.c_cflag &= ~PARENB; // 无奇偶校验
tty.c_cflag &= ~CSTOPB; // 1位停止位
tty.c_cflag &= ~CRTSCTS; // 禁用硬件流控制
if (tcsetattr(serial_fd, TCSANOW, &tty) != 0)
{
perror("无法设置串口属性");
exit(1);
}
for (int i = 0; i < array_length; i++)
{
char data[16];
sprintf(data, "%d", data_array[i]); // 将整数转换为字符串
bytes_written = write(serial_fd, data, strlen(data));
if (bytes_written < 0)
{
perror("写入串口失败");
exit(1);
}
usleep(100000); // 延时100ms
}
close(serial_fd);
return 0;
}
```
这段代码首先通过打开串口设备文件来获取串口文件描述符,然后设置串口属性,包括波特率、数据位、奇偶校验等等。接着使用循环将数组中的每个元素转换为字符串,并通过串口发送。代码中使用的是Linux系统上的串口设备地址和函数,如果在其他平台上运行,可能需要根据实际情况进行相应的修改。
相关推荐
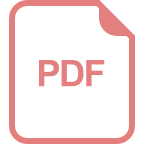
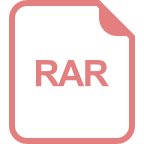
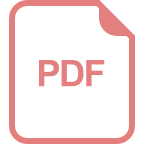
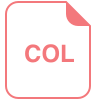
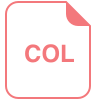
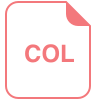
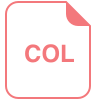










