C语言程序设计中如何输入大写字母输出小写字母
时间: 2024-09-25 21:06:43 浏览: 57
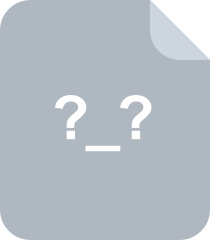
C语言程序设计-从键盘输入一个大写字母,要求改用小写字母输出;.c
在C语言中,如果你想让用户输入大写字母并将其转换为小写字母,可以借助标准库函数`tolower()`。下面是一个简单的例子:
```c
#include <stdio.h> // 包含标准输入输出头文件
#include <ctype.h> // 包含处理字符类型的头文件
int main() {
char user_input[50]; // 定义足够大的数组存储用户输入
printf("请输入一个大写字母:\n");
// 使用fgets读入用户输入,同时包含换行符
fgets(user_input, sizeof(user_input), stdin);
// 检查输入是否包含大写字母,如果不是则不做转换
for (size_t i = 0; i < strlen(user_input); ++i) {
if (isupper(user_input[i])) { // 判断字符是否为大写字母
user_input[i] = tolower(user_input[i]); // 转换成小写字母
}
}
printf("转换后的小写字母是:%s\n", user_input);
return 0;
}
```
这个程序首先提示用户输入一个大写字母,然后遍历输入的每一个字符,如果遇到大写字母,就用`tolower()`函数将其转换为小写字母。
阅读全文
相关推荐
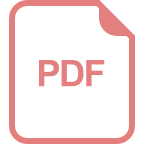
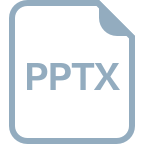
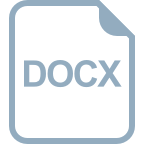
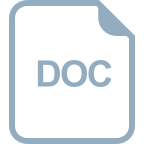











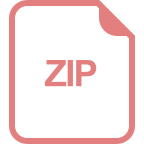