wxwidgets telnet输入存储数据 完整代码
时间: 2024-05-12 11:11:09 浏览: 237
wxWidgets是一个C++ GUI库,可以用于创建跨平台的应用程序。而Telnet是一种网络协议,可以用于在远程计算机上进行命令行操作。如果你想在wxWidgets应用程序中实现telnet输入存储数据的功能,可以参考以下示例代码:
```
#include <wx/wx.h>
#include <wx/socket.h>
class MyFrame : public wxFrame {
public:
MyFrame(const wxString& title, const wxPoint& pos, const wxSize& size);
private:
wxTextCtrl* m_textCtrl;
wxSocketClient* m_client;
wxString m_buffer;
void OnConnect(wxCommandEvent& event);
void OnSend(wxCommandEvent& event);
void OnReceive(wxSocketEvent& event);
void OnClose(wxSocketEvent& event);
wxDECLARE_EVENT_TABLE();
};
wxBEGIN_EVENT_TABLE(MyFrame, wxFrame)
EVT_MENU(wxID_CONNECT, MyFrame::OnConnect)
EVT_MENU(wxID_SEND, MyFrame::OnSend)
EVT_SOCKET(wxID_ANY, MyFrame::OnReceive)
EVT_SOCKET(wxSOCKET_LOST, MyFrame::OnClose)
wxEND_EVENT_TABLE()
MyFrame::MyFrame(const wxString& title, const wxPoint& pos, const wxSize& size)
: wxFrame(nullptr, wxID_ANY, title, pos, size), m_client(nullptr)
{
// 创建菜单栏
wxMenu* menuFile = new wxMenu;
menuFile->Append(wxID_CONNECT, "&Connect...\tCtrl-C",
"Connect to the remote server");
menuFile->Append(wxID_SEND, "&Send...\tCtrl-S",
"Send data to the remote server");
menuFile->Append(wxID_EXIT);
wxMenuBar* menuBar = new wxMenuBar;
menuBar->Append(menuFile, "&File");
SetMenuBar(menuBar);
// 创建文本控件
m_textCtrl = new wxTextCtrl(this, wxID_ANY, wxEmptyString,
wxDefaultPosition, wxDefaultSize, wxTE_MULTILINE | wxTE_READONLY);
// 布局
wxBoxSizer* sizer = new wxBoxSizer(wxVERTICAL);
sizer->Add(m_textCtrl, 1, wxEXPAND);
SetSizer(sizer);
}
void MyFrame::OnConnect(wxCommandEvent& event)
{
if (m_client) {
wxLogError("Already connected");
return;
}
wxString hostname = wxGetTextFromUser("Enter the hostname:", "Connect");
if (hostname.IsEmpty())
return;
int port = wxGetNumberFromUser("Enter the port number:", "Connect", "Port", 23, 1, 65535);
if (port == -1)
return;
// 创建客户端套接字
m_client = new wxSocketClient(wxSOCKET_BLOCK);
m_client->SetEventHandler(*this);
m_client->SetNotify(wxSOCKET_CONNECTION_FLAG | wxSOCKET_INPUT_FLAG | wxSOCKET_LOST_FLAG);
m_client->Notify(true);
// 连接到远程服务器
if (m_client->Connect(hostname, port) != wxSOCKET_NOERROR) {
delete m_client;
m_client = nullptr;
wxLogError("Failed to connect to %s:%d", hostname, port);
return;
}
m_textCtrl->AppendText("Connected to " + hostname + ":" + wxString::Format("%d", port) + "\n");
}
void MyFrame::OnSend(wxCommandEvent& event)
{
if (!m_client) {
wxLogError("Not connected");
return;
}
wxString data = wxGetTextFromUser("Enter the data to send:", "Send");
if (data.IsEmpty())
return;
// 发送数据到远程服务器
if (m_client->Write(data) != wxSOCKET_NOERROR) {
wxLogError("Failed to send data");
return;
}
m_textCtrl->AppendText("Sent: " + data + "\n");
}
void MyFrame::OnReceive(wxSocketEvent& event)
{
if (!m_client)
return;
if (event.GetSocket() != m_client)
return;
// 从套接字中读取数据
char buffer[1024];
size_t len = m_client->Read(buffer, sizeof(buffer));
if (len == 0) {
OnClose(event);
return;
}
// 将数据存储在缓冲区中
m_buffer += wxString(buffer, len);
// 按行分割数据
size_t pos = 0;
while ((pos = m_buffer.find("\n")) != wxString::npos) {
wxString line = m_buffer.SubString(0, pos);
m_buffer = m_buffer.Mid(pos + 1);
// 将行写入文本控件中
m_textCtrl->AppendText("Received: " + line + "\n");
}
}
void MyFrame::OnClose(wxSocketEvent& event)
{
if (!m_client)
return;
if (event.GetSocket() != m_client)
return;
// 关闭套接字并删除客户端对象
m_client->Destroy();
m_client = nullptr;
m_textCtrl->AppendText("Connection closed\n");
}
class MyApp : public wxApp {
public:
virtual bool OnInit();
};
bool MyApp::OnInit()
{
MyFrame* frame = new MyFrame("Telnet Client", wxDefaultPosition, wxSize(640, 480));
frame->Show();
return true;
}
wxIMPLEMENT_APP(MyApp);
```
这段代码实现了一个简单的telnet客户端,它可以连接到远程服务器,并将用户输入的数据发送到服务器。收到的数据将被按行分割,并存储在缓冲区中。当完整的行被接收时,它将被写入文本控件中。你可以根据自己的需要修改代码,并将它集成到你的wxWidgets应用程序中。
阅读全文
相关推荐
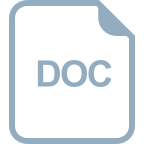
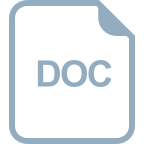
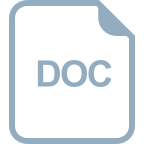

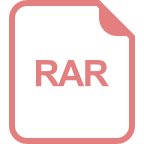
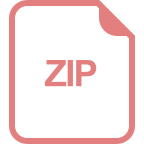

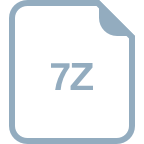
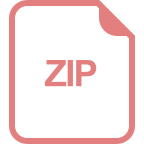
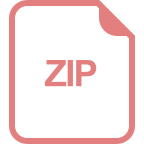
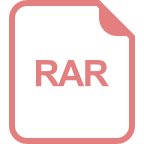
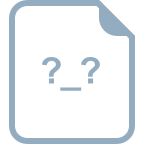
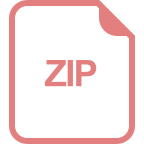
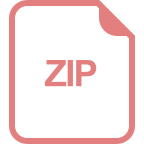
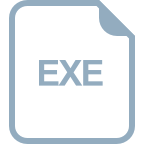


