python弹球游戏详细设计
时间: 2023-07-25 11:11:56 浏览: 44
Python 弹球游戏的设计可以分为以下几个步骤:
1. 导入必要的库
```python
import pygame
import random
import math
```
2. 初始化 Pygame
```python
pygame.init()
# 设置窗口大小
screen = pygame.display.set_mode((800, 600))
# 设置游戏标题
pygame.display.set_caption("弹球游戏")
# 设置游戏时钟
clock = pygame.time.Clock()
```
3. 定义游戏对象
```python
# 定义球的属性
ball_image = pygame.image.load("ball.png")
ball_rect = ball_image.get_rect()
ball_speed = 5
ball_direction = random.randint(0, 360)
# 定义挡板的属性
paddle_image = pygame.image.load("paddle.png")
paddle_rect = paddle_image.get_rect()
paddle_speed = 5
# 定义砖块的属性
brick_images = []
brick_rects = []
brick_rows = 5
brick_cols = 10
brick_width = 70
brick_height = 30
brick_gap = 10
brick_colors = [(255, 0, 0), (255, 255, 0), (0, 255, 0), (0, 0, 255), (255, 0, 255)]
for row in range(brick_rows):
for col in range(brick_cols):
brick_image = pygame.Surface((brick_width, brick_height))
brick_image.fill(brick_colors[row])
brick_rect = brick_image.get_rect()
brick_rect.x = col * (brick_width + brick_gap)
brick_rect.y = row * (brick_height + brick_gap)
brick_images.append(brick_image)
brick_rects.append(brick_rect)
```
4. 定义游戏循环
```python
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 移动球
ball_direction_rad = math.radians(ball_direction)
ball_rect.x += ball_speed * math.cos(ball_direction_rad)
ball_rect.y += ball_speed * math.sin(ball_direction_rad)
# 碰撞检测
if ball_rect.left < 0 or ball_rect.right > screen.get_width():
ball_direction = (180 - ball_direction) % 360
if ball_rect.top < 0:
ball_direction = (360 - ball_direction) % 360
if ball_rect.bottom > screen.get_height():
ball_rect.center = screen.get_rect().center
ball_direction = random.randint(0, 360)
if ball_rect.colliderect(paddle_rect):
ball_direction = (360 - ball_direction) % 360
for brick_rect in brick_rects:
if ball_rect.colliderect(brick_rect):
ball_direction = (360 - ball_direction) % 360
brick_rects.remove(brick_rect)
brick_images.remove(brick_images[brick_rects.index(brick_rect)])
# 移动挡板
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT] and paddle_rect.left > 0:
paddle_rect.x -= paddle_speed
if keys[pygame.K_RIGHT] and paddle_rect.right < screen.get_width():
paddle_rect.x += paddle_speed
# 绘制游戏界面
screen.fill((255, 255, 255))
for brick_image, brick_rect in zip(brick_images, brick_rects):
screen.blit(brick_image, brick_rect)
screen.blit(ball_image, ball_rect)
screen.blit(paddle_image, paddle_rect)
# 更新游戏界面
pygame.display.update()
# 控制游戏帧率
clock.tick(60)
```
5. 运行游戏
```python
if __name__ == "__main__":
main()
```
以上就是 Python 弹球游戏的详细设计。需要注意的是,以上代码仅作为参考,实际开发中还需要根据自己的需求进行适当的修改和优化。
相关推荐
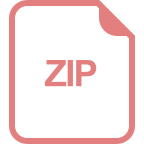
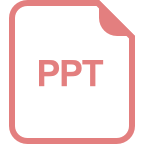








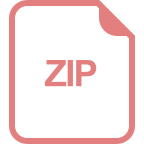
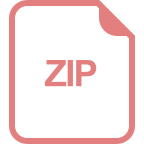
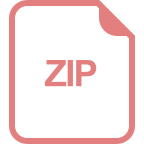
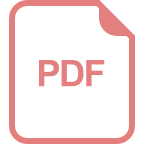
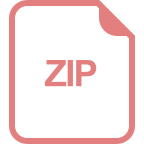
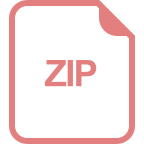
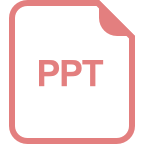