深度学习图像修复pytorch代码
时间: 2024-10-14 18:15:39 浏览: 34
深度学习图像修复通常使用生成式对抗网络 (GANs) 或者基于自编码器的模型,如PixelCNN、CycleGAN等。在PyTorch中,你可以采用U-Net这样的卷积神经网络架构来进行图像修复任务。下面是一个简单的U-Net示例代码片段:
```python
import torch
import torch.nn as nn
# 定义U-Net结构
class UNet(nn.Module):
def __init__(self, in_channels=3, out_channels=3, depth=5, start_filts=64):
super(UNet, self).__init__()
# 编码部分
encoder_down = []
for _ in range(depth):
encoder_down.append(
nn.Sequential(
nn.Conv2d(in_channels, start_filts * (2 ** (_ + 1)), kernel_size=4, stride=2, padding=1),
nn.BatchNorm2d(start_filts * (2 ** (_ + 1))),
nn.ReLU(True)
)
)
in_channels = start_filts * (2 ** (_ + 1))
# 中间层
self.center = nn.Sequential(
nn.Conv2d(in_channels, start_filts * (2 ** (depth + 1)), kernel_size=3, padding=1),
nn.BatchNorm2d(start_filts * (2 ** (depth + 1))),
nn.ReLU(True)
)
# 解码部分
decoder_up = []
for _ in range(depth):
decoder_up.append(
nn.Sequential(
nn.ConvTranspose2d(start_filts * (2 ** (depth - _)), start_filts * (2 ** (_ + 1)) // 2, kernel_size=4, stride=2, padding=1),
nn.BatchNorm2d(start_filts * (2 ** (_ + 1)) // 2),
nn.ReLU(True),
nn.Conv2d(start_filts * (2 ** (_ + 1)) // 2, start_filts * (2 ** (_ + 1)) // 2, kernel_size=3, padding=1),
nn.BatchNorm2d(start_filts * (2 ** (_ + 1)) // 2),
nn.ReLU(True)
)
)
decoder_up.append(nn.ConvTranspose2d(start_filts, out_channels, kernel_size=4, stride=2, padding=1))
self.decoder_up = nn.ModuleList(decoder_up)
self.encoder_down = nn.ModuleList(encoder_down)
def forward(self, x):
encoder_outputs = []
for enc in self.encoder_down:
x = enc(x)
encoder_outputs.append(x)
center = self.center(x)
decoder_output = self.decoder_up[0](center)
for i in range(1, len(self.decoder_up)):
decoder_output = torch.cat([decoder_output, encoder_outputs.pop()], dim=1)
decoder_output = self.decoder_up[i](decoder_output)
return decoder_output
# 使用示例
model = UNet()
input_image = torch.randn(1, 3, 256, 256)
output = model(input_image)
```
阅读全文
相关推荐
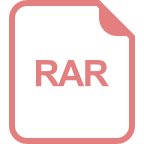
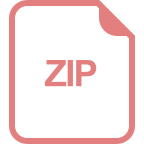
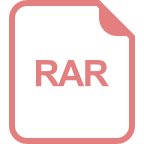















