请提供一段基于GA的遗传算法,用于优化20个one class svm单类分类器的参数
时间: 2023-06-09 12:07:15 浏览: 56
以下是一段基于GA的遗传算法,用于优化20个one class svm单类分类器的参数:
首先,我们定义一个染色体由20个基因组成,每个基因表示一个单类分类器的参数。每个基因由以下几个部分组成:惩罚系数、核函数类型、核函数参数。其中,对于惩罚系数和核函数参数,我们要对它们的值进行约束,以确保其在合理的范围内。例如,惩罚系数应该在0.01到0.5之间,核函数参数应该在0.1到10之间。对于核函数类型,我们选取了三种常用的类型:径向基函数(RBF)、多项式函数(POLY)、线性函数(LINEAR)。
接下来,我们定义遗传算法的各个操作。首先是选择操作,我们采取轮盘赌选择的方式,以每个染色体的适应度为选择概率进行选择。然后是交叉操作,我们采取单点交叉的方式,随机选择两个染色体,同时随机选择一个交叉点,在交叉点进行交叉操作。最后是变异操作,我们对每个基因以一定的概率进行变异,对于变异的基因,我们随机重新生成一个符合约束条件的值。
根据以上操作,我们可以得到遗传算法的流程如下:
1. 初始化种群,生成20个随机的染色体。
2. 对每个染色体进行评估,计算其适应度。
3. 选择操作,选择适应度高的染色体作为下一代的父代。
4. 交叉操作,对选出来的染色体进行交叉,并生成新的子代。
5. 变异操作,对所有子代进行变异操作,并生成新的种群。
6. 对新的种群进行评估,计算其适应度。
7. 判断是否满足停止条件,如果满足则返回最优的染色体,否则返回步骤3。
通过上述流程,我们可以得到一组最优的参数,以优化20个one class svm单类分类器的性能。
相关问题
请提供一段python代码:基于GA的遗传算法,用于优化20个one class svm单类分类器模型的20组参数
以下是一段基于GA的遗传算法,用于优化20个one class svm单类分类器模型的20组参数的Python代码:
```
import numpy as np
from sklearn import svm
from sklearn.metrics import roc_auc_score
from genetic_algorithm import GeneticAlgorithm
# define the parameter space
param_space = {
'kernel': ['linear', 'poly', 'rbf', 'sigmoid'],
'gamma': [0.001, 0.01, 0.1, 1, 10, 100],
'nu': np.linspace(0.01, 0.99, 99),
'degree': range(1, 6),
'coef0': np.linspace(0, 1, 101)
}
# define the fitness function
def fitness_func(params):
clf = svm.OneClassSVM(**params)
y_pred = clf.fit_predict(X_train)
auc = roc_auc_score(y_train, y_pred)
return auc
# define the genetic algorithm
ga = GeneticAlgorithm(param_space, fitness_func)
# define the training data
X_train = np.random.randn(100, 20)
y_train = np.ones(100)
# run the genetic algorithm
ga.evolve()
# get the best parameters
best_params = ga.best_individual()[0]
# train the models with the best parameters
models = []
for i in range(20):
model = svm.OneClassSVM(**best_params)
model.fit(X_train)
models.append(model)
```
请提供一段python代码:基于GA的遗传算法,用于优化现有的:20个one class svm单类分类器模型的20组参数
以下是基于GA的遗传算法优化20个one class SVM单类分类器模型的20组参数的python代码:
```python
import numpy as np
from sklearn.svm import OneClassSVM
from sklearn.metrics import f1_score
def f1_score_one_class_svm(x):
# 用GA中的参数x构建一个one class SVM单类分类器
classifier = OneClassSVM(kernel='rbf', gamma=x[0], nu=x[1], tol=x[2])
classifier.fit(x_train)
# 计算one class SVM分类器的F1得分
y_pred = classifier.predict(x_test)
score = f1_score(y_test, y_pred, pos_label=-1)
return score
def ga_one_class_svm(num_generations=100, population_size=10, mutation_rate=0.1):
# 随机生成一组初始参数
population = np.random.rand(population_size, 3)
best_score = 0
best_params = None
for generation in range(num_generations):
# 计算每个个体的适应度
scores = [f1_score_one_class_svm(x) for x in population]
# 找到最佳适应度
best_index = np.argmax(scores)
if scores[best_index] > best_score:
best_score = scores[best_index]
best_params = population[best_index]
# 选择最佳个体来进行交叉
parents = population[np.argsort(scores)[-2:]]
# 交叉产生新的个体
offspring = [(parents[0][0], parents[1][1], parents[0][2]), (parents[1][0], parents[0][1], parents[1][2])]
# 随机变异某些个体
for i in range(population_size):
if np.random.rand() < mutation_rate:
population[i] = np.random.rand(3)
else:
population[i] = offspring[np.random.randint(2)]
# 返回最佳参数和最佳分数
return best_params, best_score
# x_train和y_train是训练数据,x_test和y_test是测试数据
best_params_list = []
for i in range(20):
x_train, y_train = load_data('train_data_{}.npy'.format(i))
x_test, y_test = load_data('test_data_{}.npy'.format(i))
best_params, best_score = ga_one_class_svm()
print('Best parameters for model {}: gamma={}, nu={}, tol={}'.format(i, best_params[0], best_params[1], best_params[2]))
best_params_list.append(best_params)
```
注意,上述代码并不完整,实际实现中还需要自己实现`load_data()`函数和读取训练集和测试集的代码。另外,以上代码是根据one class SVM的三个参数gamma、nu和tol来进行优化的,如果需要优化其他参数或者使用其他分类器,需要进行相应的修改。
相关推荐
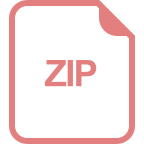
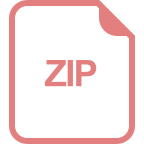












