用c语言写一个哈希表例子
时间: 2024-05-06 17:16:19 浏览: 27
好的,下面是一个简单的哈希表实现的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define TABLE_SIZE 100
typedef struct Node {
char *key;
char *value;
struct Node *next;
} Node;
Node *hash_table[TABLE_SIZE];
// 哈希函数,将字符串转换为数字下标
int hash_function(char *key) {
int sum = 0;
while (*key) {
sum += *key++;
}
return sum % TABLE_SIZE;
}
// 插入键值对
void insert(char *key, char *value) {
Node *node = (Node *) malloc(sizeof(Node));
node->key = key;
node->value = value;
int index = hash_function(key);
node->next = hash_table[index];
hash_table[index] = node;
}
// 查找键对应的值
char *search(char *key) {
int index = hash_function(key);
Node *node = hash_table[index];
while (node) {
if (strcmp(node->key, key) == 0) {
return node->value;
}
node = node->next;
}
return NULL;
}
int main() {
// 插入一些键值对
insert("apple", "red");
insert("banana", "yellow");
insert("orange", "orange");
insert("grape", "purple");
// 查找键对应的值
printf("%s\n", search("apple")); // 输出 "red"
printf("%s\n", search("banana")); // 输出 "yellow"
printf("%s\n", search("orange")); // 输出 "orange"
printf("%s\n", search("grape")); // 输出 "purple"
printf("%s\n", search("pear")); // 输出 "(null)"
return 0;
}
```
以上代码实现了一个简单的哈希表,可以插入键值对并查找键对应的值。在这个例子中,我们使用了一个数组来存储哈希表,每个元素都是一个链表头指针。当插入一个新的键值对时,首先根据键计算出其在数组中的下标,然后将该键值对插入到对应链表的头部。查找键对应的值时,同样需要先计算出其在数组中的下标,然后遍历对应链表,查找是否存在该键对应的节点。
阅读全文
相关推荐
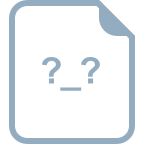


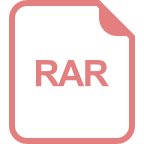


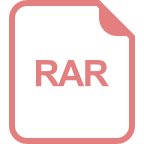
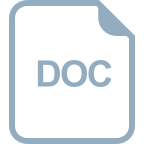
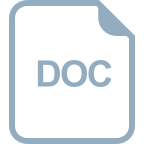


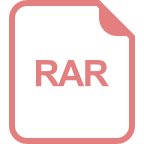
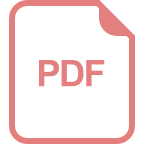
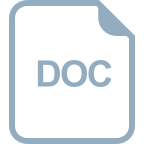


