用 C 语言实现 LFU 算法
时间: 2023-06-05 07:06:40 浏览: 110
很高兴回答你的问题。LFU(Least Frequently Used)算法是一种缓存淘汰算法,用于在内存中缓存最近使用频率最低的数据。要使用 C 语言实现 LFU 算法,需要使用数据结构,如哈希表或双向链表,来快速访问缓存中的数据,并使用计数器来跟踪每个数据项的使用频率。实现过程包括以下步骤:
1. 初始化哈希表或双向链表,其中包含缓存的数据项;
2. 创建一个计数器数组,用于记录每个数据项的使用频率;
3. 当有新的数据项插入缓存时,将其插入哈希表或双向链表中,并将其使用频率记录为1;
4. 当缓存达到最大容量时,删除使用频率最低的数据项,即计数器数组中的最小值;
5. 每次访问缓存中的数据项时,将其使用频率加1,并更新计数器数组;
6. 如果缓存中存在多个使用频率相同的数据项,删除最先进入缓存的数据项。
希望对你有所帮助。
相关问题
lfu算法c语言
以下是 C 语言实现的 LFU 算法示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define CACHE_SIZE 5
typedef struct Node {
int key;
int value;
int freq;
struct Node *prev;
struct Node *next;
} Node;
typedef struct {
Node *head;
Node *tail;
} List;
typedef struct {
int size;
int capacity;
List *freq_list;
Node **cache;
} LFUCache;
Node *create_node(int key, int value) {
Node *node = (Node *)malloc(sizeof(Node));
node->key = key;
node->value = value;
node->freq = 1;
node->prev = NULL;
node->next = NULL;
return node;
}
List *create_list() {
List *list = (List *)malloc(sizeof(List));
list->head = NULL;
list->tail = NULL;
return list;
}
LFUCache *create_cache(int capacity) {
LFUCache *cache = (LFUCache *)malloc(sizeof(LFUCache));
cache->size = 0;
cache->capacity = capacity;
cache->freq_list = (List *)malloc(sizeof(List) * (CACHE_SIZE + 1));
cache->cache = (Node **)calloc(capacity, sizeof(Node *));
return cache;
}
void delete_node(LFUCache *cache, Node *node) {
List *list = &cache->freq_list[node->freq];
if (node->prev == NULL) {
list->head = node->next;
} else {
node->prev->next = node->next;
}
if (node->next == NULL) {
list->tail = node->prev;
} else {
node->next->prev = node->prev;
}
cache->size--;
free(node);
}
void insert_node(LFUCache *cache, Node *node) {
List *list = &cache->freq_list[node->freq];
if (list->head == NULL) {
list->head = node;
list->tail = node;
} else {
node->next = list->head;
list->head->prev = node;
list->head = node;
}
cache->size++;
}
void update_node(LFUCache *cache, Node *node) {
delete_node(cache, node);
node->freq++;
insert_node(cache, node);
}
void remove_least_frequent_node(LFUCache *cache) {
int i = 1;
List *list;
while (i <= CACHE_SIZE && (list = &cache->freq_list[i])->head == NULL) {
i++;
}
if (i > CACHE_SIZE) {
return;
}
Node *node = list->tail;
delete_node(cache, node);
cache->cache[node->key] = NULL;
}
int get(LFUCache *cache, int key) {
if (cache->capacity == 0) {
return -1;
}
Node *node = cache->cache[key];
if (node == NULL) {
return -1;
}
update_node(cache, node);
return node->value;
}
void put(LFUCache *cache, int key, int value) {
if (cache->capacity == 0) {
return;
}
Node *node = cache->cache[key];
if (node == NULL) {
if (cache->size == cache->capacity) {
remove_least_frequent_node(cache);
}
node = create_node(key, value);
cache->cache[key] = node;
insert_node(cache, node);
} else {
node->value = value;
update_node(cache, node);
}
}
int main() {
LFUCache *cache = create_cache(2);
put(cache, 1, 1);
put(cache, 2, 2);
printf("%d\n", get(cache, 1)); // 1
put(cache, 3, 3);
printf("%d\n", get(cache, 2)); // -1
printf("%d\n", get(cache, 3)); // 3
put(cache, 4, 4);
printf("%d\n", get(cache, 1)); // -1
printf("%d\n", get(cache, 3)); // 3
printf("%d\n", get(cache, 4)); // 4
return 0;
}
```
该示例中,LFUCache 结构体表示 LFU 缓存,它包含了缓存的大小、容量、频率列表和缓存数组。Node 结构体表示缓存中的节点,它包含了键、值、频率以及前驱和后继节点。List 结构体表示频率列表,它包含了头节点和尾节点。create_node() 函数用于创建节点,create_list() 函数用于创建列表,create_cache() 函数用于创建缓存。delete_node() 函数用于删除节点,insert_node() 函数用于插入节点,update_node() 函数用于更新节点,remove_least_frequent_node() 函数用于删除最不经常使用的节点。get() 函数用于获取缓存中指定键的值,put() 函数用于向缓存中插入指定键值对。
示例中的 put() 函数实现比较复杂,需要分别处理插入新节点和更新已有节点的情况,还需要删除最不经常使用的节点以腾出空间。因此,put() 函数的时间复杂度为 O(log n),其中 n 为缓存的容量。get() 函数的实现相对简单,只需要更新节点的频率即可,因此时间复杂度为 O(1)。
实现最不经常使用算法(LFU)代码c语言
LFU(Least Frequently Used)算法是一种缓存淘汰算法,它根据缓存中每个条目的使用频率来选择要淘汰的条目。以下是一个简单的 LFU 算法的 C 语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int key;
int value;
int freq;
struct node* prev;
struct node* next;
} Node;
typedef struct {
int size;
int capacity;
Node* head;
Node* tail;
Node** freqList;
} LFUCache;
Node* createNode(int key, int value) {
Node* node = (Node*) malloc(sizeof(Node));
node->key = key;
node->value = value;
node->freq = 1;
node->prev = NULL;
node->next = NULL;
return node;
}
LFUCache* createLFUCache(int capacity) {
LFUCache* cache = (LFUCache*) malloc(sizeof(LFUCache));
cache->size = 0;
cache->capacity = capacity;
cache->head = NULL;
cache->tail = NULL;
cache->freqList = (Node**) calloc(capacity + 1, sizeof(Node*));
return cache;
}
void deleteNode(LFUCache* cache, Node* node) {
if (node == cache->head) {
cache->head = node->next;
} else if (node == cache->tail) {
cache->tail = node->prev;
cache->tail->next = NULL;
} else {
node->prev->next = node->next;
node->next->prev = node->prev;
}
cache->size--;
cache->freqList[node->freq] = NULL;
free(node);
}
void insertNode(LFUCache* cache, Node* node) {
if (cache->head == NULL) {
cache->head = node;
cache->tail = node;
} else {
node->next = cache->head;
cache->head->prev = node;
cache->head = node;
}
cache->size++;
cache->freqList[node->freq] = node;
}
int get(LFUCache* cache, int key) {
Node* node = cache->freqList[key];
if (node == NULL) {
return -1;
}
node->freq++;
if (node != cache->head) {
deleteNode(cache, node);
insertNode(cache, node);
}
return node->value;
}
void put(LFUCache* cache, int key, int value) {
if (cache->capacity == 0) {
return;
}
Node* node = cache->freqList[key];
if (node != NULL) {
node->value = value;
node->freq++;
if (node != cache->head) {
deleteNode(cache, node);
insertNode(cache, node);
}
} else {
node = createNode(key, value);
if (cache->size == cache->capacity) {
Node* tail = cache->tail;
deleteNode(cache, tail);
}
insertNode(cache, node);
}
}
void printCache(LFUCache* cache) {
Node* node = cache->head;
while (node != NULL) {
printf("(%d:%d:%d) ", node->key, node->value, node->freq);
node = node->next;
}
printf("\n");
}
int main() {
LFUCache* cache = createLFUCache(2);
put(cache, 1, 1);
put(cache, 2, 2);
printCache(cache);
printf("%d\n", get(cache, 1));
printCache(cache);
put(cache, 3, 3);
printCache(cache);
printf("%d\n", get(cache, 2));
printf("%d\n", get(cache, 3));
put(cache, 4, 4);
printf("%d\n", get(cache, 1));
printf("%d\n", get(cache, 3));
printf("%d\n", get(cache, 4));
printCache(cache);
return 0;
}
```
在这个实现中,LFUCache 结构体维护了缓存的大小和容量,以及缓存中每个访问频率的节点。Node 结构体表示缓存中的每个元素。createNode 函数用于创建新节点,insertNode 函数用于将节点插入到缓存中,deleteNode 函数用于从缓存中删除节点,get 函数用于获取缓存中指定键的值,并将节点的访问频率加一,put 函数用于将新元素放入缓存中。最后,printCache 函数用于打印缓存中的所有元素。
在 main 函数中,我们创建一个大小为 2 的 LFU 缓存,向其中插入两个元素,打印缓存中的所有元素,然后获取键为 1 的元素,再次打印缓存中的所有元素,向缓存中插入一个新元素,打印缓存中的所有元素,获取键为 2 和 3 的元素,向缓存中插入一个新元素,并打印缓存中的所有元素。
相关推荐
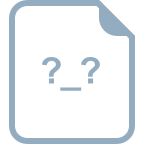
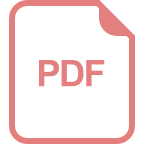








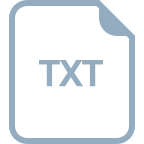