用Python写出BP神经网络求函数极值的代码
时间: 2023-11-19 15:59:01 浏览: 83
以下是使用Python编写BP神经网络求函数极值的代码示例:
```python
import numpy as np
# 激活函数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 求导数
def derivative_sigmoid(x):
return sigmoid(x) * (1 - sigmoid(x))
# BP神经网络
class BPNeuralNetwork():
def __init__(self):
np.random.seed(1)
self.weights = 2 * np.random.random((1, 4)) - 1
self.bias = 2 * np.random.random((1, 1)) - 1
def train(self, inputs, outputs, iterations):
for iteration in range(iterations):
# 前向传播
layer_1 = sigmoid(np.dot(inputs, self.weights) + self.bias)
# 反向传播
error = outputs - layer_1
adjust = error * derivative_sigmoid(layer_1)
self.weights += np.dot(inputs.T, adjust)
self.bias += np.sum(adjust, axis=0, keepdims=True)
def predict(self, inputs):
return sigmoid(np.dot(inputs, self.weights) + self.bias)
# 测试代码
if __name__ == "__main__":
# 设置参数
X = np.array([[0, 0, 1], [0, 1, 1], [1, 0, 1], [1, 1, 1]])
y = np.array([[0, 0, 1, 1]]).T
iterations = 10000
learning_rate = 0.1
# 训练模型
nn = BPNeuralNetwork()
nn.train(X, y, iterations)
# 预测结果
test_inputs = np.array([[1, 1, 0]])
print("Predicted Output: ", nn.predict(test_inputs))
```
应该注意的是,这只是一个简单的示例。在实际应用中,可能需要使用更复杂的网络结构和算法来解决更复杂的问题。
阅读全文
相关推荐
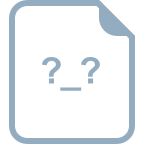
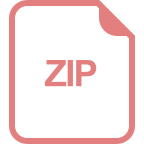
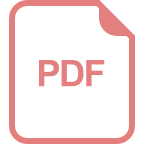
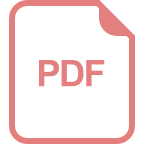
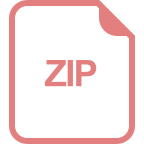
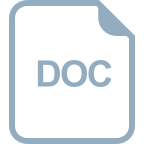
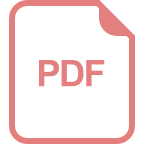
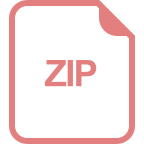
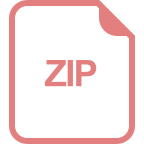
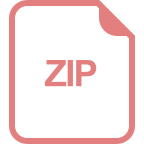
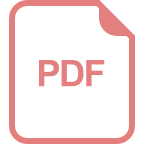
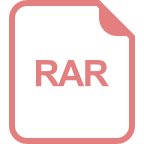
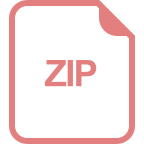