为这段代码添加中文释义import heapq from collections import defaultdict def huffman_encoding(data): # 计算字符频率 freq = defaultdict(int) for char in data: freq[char] += 1 # 将频率转化为堆 heap = [[weight, [char, ""]] for char, weight in freq.items()] heapq.heapify(heap) # 合并堆中的节点,生成霍夫曼编码树 while len(heap) > 1: low = heapq.heappop(heap) high = heapq.heappop(heap) for pair in low[1:]: pair[1] = '0' + pair[1] for pair in high[1:]: pair[1] = '1' + pair[1] heapq.heappush(heap, [low[0] + high[0]] + low[1:] + high[1:]) # 生成霍夫曼编码表 code_table = dict(heapq.heappop(heap)[1:]) # 编码 encoded_data = "" for char in data: encoded_data += code_table[char] return encoded_data, code_table def huffman_decoding(encoded_data, code_table): # 将编码表反转,方便解码 reverse_code_table = {v: k for k, v in code_table.items()} # 解码 decoded_data = "" code = "" for bit in encoded_data: code += bit if code in reverse_code_table: decoded_data += reverse_code_table[code] code = "" return decoded_data data = "hello world" encoded_data, code_table = huffman_encoding(data) print("Encoded data:", encoded_data) print("Code table:", code_table) decoded_data = huffman_decoding(encoded_data, code_table) print("Decoded data:", decoded_data)
时间: 2024-03-03 20:51:41 浏览: 154
```python
import heapq
from collections import defaultdict
def huffman_encoding(data):
# 计算字符频率
freq = defaultdict(int) # 使用 defaultdict 来实现字符频率的计算
for char in data:
freq[char] += 1
# 将频率转化为堆
heap = [[weight, [char, ""]] for char, weight in freq.items()] # 将频率转化为堆
heapq.heapify(heap)
# 合并堆中的节点,生成霍夫曼编码树
while len(heap) > 1:
low = heapq.heappop(heap)
high = heapq.heappop(heap)
for pair in low[1:]:
pair[1] = '0' + pair[1]
for pair in high[1:]:
pair[1] = '1' + pair[1]
heapq.heappush(heap, [low[0] + high[0]] + low[1:] + high[1:])
# 生成霍夫曼编码表
code_table = dict(heapq.heappop(heap)[1:]) # 生成霍夫曼编码表
# 编码
encoded_data = ""
for char in data:
encoded_data += code_table[char] # 编码数据
return encoded_data, code_table
def huffman_decoding(encoded_data, code_table):
# 将编码表反转,方便解码
reverse_code_table = {v: k for k, v in code_table.items()} # 将编码表反转,方便解码
# 解码
decoded_data = ""
code = ""
for bit in encoded_data:
code += bit
if code in reverse_code_table:
decoded_data += reverse_code_table[code]
code = ""
return decoded_data
data = "hello world"
encoded_data, code_table = huffman_encoding(data)
print("Encoded data:", encoded_data)
print("Code table:", code_table)
decoded_data = huffman_decoding(encoded_data, code_table)
print("Decoded data:", decoded_data)
```
注释已添加,方便理解代码的实现过程。
阅读全文
相关推荐
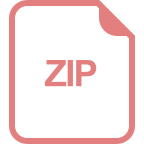
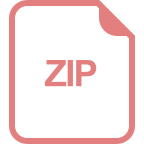
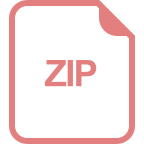

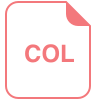













