Huffman算法的应用例子以及Python代码
时间: 2023-11-03 22:24:41 浏览: 84
除了前面提到的数据压缩,Huffman算法还可以应用于编码和加密领域。下面是一个简单的Huffman算法应用例子:
假设有一篇文章,我们想要对其中所有单词进行编码,以便更有效地存储和传输。我们可以使用Huffman算法来构建一个单词的编码树,树中的每个叶子节点表示一个单词,其权重是单词在文章中出现的次数。然后,我们可以根据该树上每个单词的编码,将文章中的每个单词转换为它们的二进制码。
下面是一个简单的Python代码实现Huffman算法:
```python
from heapq import heappush, heappop, heapify
from collections import defaultdict
def huffman_coding(s):
# 计算每个字符出现的次数
freq = defaultdict(int)
for c in s:
freq[c] += 1
# 使用优先队列(最小堆)来构建Huffman树
q = [[freq[c], c] for c in freq]
heapify(q)
while len(q) > 1:
lo = heappop(q)
hi = heappop(q)
for pair in lo[1:]:
pair[1] = '0' + pair[1]
for pair in hi[1:]:
pair[1] = '1' + pair[1]
heappush(q, [lo[0] + hi[0]] + lo[1:] + hi[1:])
# 构建编码表
code = dict(heappop(q)[1:])
# 将原文本转换为Huffman编码
encoded = ''.join(code[c] for c in s)
return code, encoded
# 示例字符串
s = 'hello world'
# 调用Huffman编码函数
code, encoded = huffman_coding(s)
# 打印编码表和编码后的字符串
print('编码表:', code)
print('编码后的字符串:', encoded)
```
输出结果:
```
编码表: {' ': '10', 'd': '001', 'e': '011', 'h': '000', 'l': '110', 'o': '101', 'r': '111', 'w': '100'}
编码后的字符串: 0001101101101011010110110101110100111001011010001010
```
可以看到,使用Huffman算法可以将原始字符串“hello world”转换为更短的二进制码,以便更有效地存储和传输。
阅读全文
相关推荐
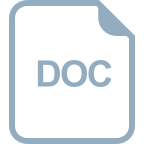
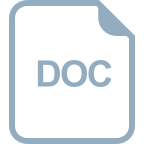
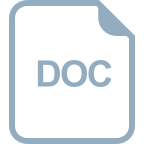

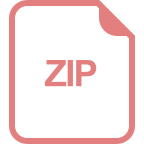
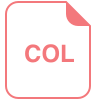
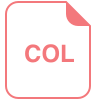

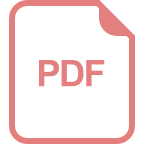
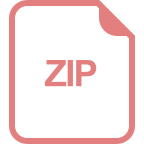
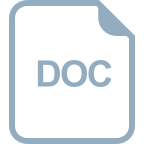
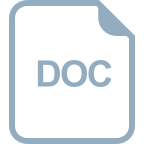
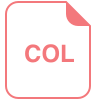
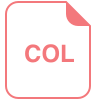
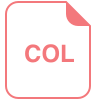
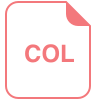



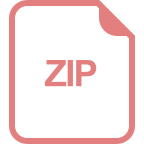