python pyglet 渲染纹理到立方体
时间: 2023-06-09 15:03:24 浏览: 200
我了解您的问题。要在Pyglet中将纹理渲染到立方体上,您需要使用OpenGL。您需要创建一个OpenGL上下文,并将其与Pyglet窗口绑定。然后,您可以使用Pyglet的Batch和Group来创建立方体,并将纹理与其关联。具体实现可以参考以下代码:
```python
import pyglet
from pyglet.gl import *
# 创建OpenGL上下文并绑定到Pyglet窗口
window = pyglet.window.Window()
context = window.context
context.set_current()
glEnable(GL_DEPTH_TEST)
# 创建Batch和Group
batch = pyglet.graphics.Batch()
group = pyglet.graphics.TextureGroup(pyglet.image.load('texture.png').texture)
# 创建立方体的顶点和纹理坐标
vertices = [
# front face
(-1, -1, 1),
( 1, -1, 1),
( 1, 1, 1),
(-1, 1, 1),
# back face
(-1, -1, -1),
(-1, 1, -1),
( 1, 1, -1),
( 1, -1, -1),
# top face
(-1, 1, -1),
(-1, 1, 1),
( 1, 1, 1),
( 1, 1, -1),
# bottom face
(-1, -1, -1),
( 1, -1, -1),
( 1, -1, 1),
(-1, -1, 1),
# left face
(-1, -1, -1),
(-1, -1, 1),
(-1, 1, 1),
(-1, 1, -1),
# right face
( 1, -1, 1),
( 1, -1, -1),
( 1, 1, -1),
( 1, 1, 1),
]
tex_coords = [
# front face
(0, 0),
(1, 0),
(1, 1),
(0, 1),
# back face
(0, 0),
(1, 0),
(1, 1),
(0, 1),
# top face
(0, 0),
(1, 0),
(1, 1),
(0, 1),
# bottom face
(0, 0),
(1, 0),
(1, 1),
(0, 1),
# left face
(0, 0),
(1, 0),
(1, 1),
(0, 1),
# right face
(0, 0),
(1, 0),
(1, 1),
(0, 1),
]
# 创建立方体
faces = [
(0, 1, 2, 3),
(4, 5, 6, 7),
(8, 9, 10, 11),
(12, 13, 14, 15),
(16, 17, 18, 19),
(20, 21, 22, 23),
]
for i, face in enumerate(faces):
vertices = []
tex_coords = []
for j in face:
vertices.extend(vertices_list[j])
tex_coords.extend(tex_coords_list[j])
batch.add_indexed(4, GL_TRIANGLE_FAN, group, range(4),
('v3f', vertices), ('t2f', tex_coords))
# 绘制立方体
@window.event
def on_draw():
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT)
glLoadIdentity()
glTranslatef(0, 0, -5)
glRotatef(30, 1, 0, 0)
glRotatef(30, 0, 1, 0)
batch.draw()
pyglet.app.run()
```
这里假设您已经有一个名为“texture.png”的纹理图像。我希望这可以帮助您解决问题。
阅读全文
相关推荐
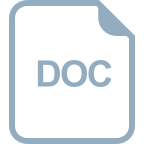
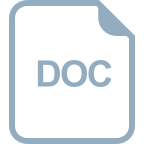
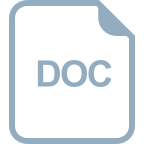
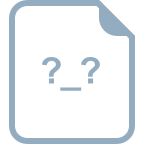
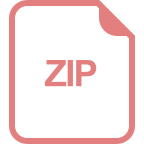
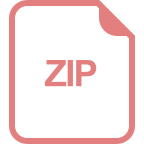
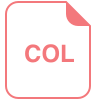
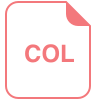
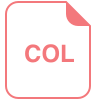
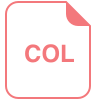

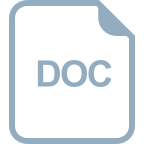
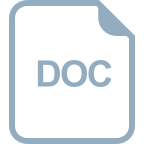
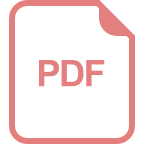
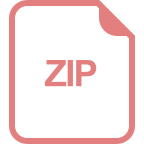
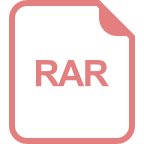
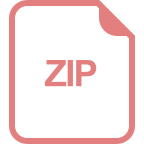
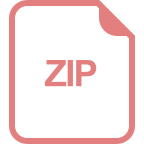
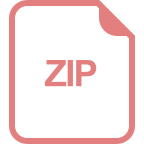