帮我找一份c++客房管理系统
时间: 2023-06-06 16:05:50 浏览: 52
好的,我可以帮您找到一些现成的 C 客房管理系统。您可以通过访问一些软件下载网站如CSDN、GitHub等找到适合您的C客房管理系统。如果您需要定制化的话,可以联系专业的软件开发公司,他们会根据您的具体需求开发出符合您要求的C客房管理系统。
相关问题
帮我写一个c++的图书管理系统
好的,下面是一个简单的C++图书管理系统的示例代码。需要注意的是,这只是一个简单的示例,可以根据实际需求进行修改和补充。
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 定义书籍结构体
struct Book {
string name; // 书名
string author; // 作者
string publisher; // 出版社
int publish_year; // 出版年份
int stock; // 库存
};
// 定义图书馆类
class Library {
public:
// 添加书籍
void add_book(string name, string author, string publisher, int publish_year, int stock) {
Book book = { name, author, publisher, publish_year, stock };
books.push_back(book);
}
// 借出书籍
void borrow_book(string name) {
for (auto& book : books) {
if (book.name == name) {
if (book.stock > 0) {
book.stock--;
cout << "借出成功!" << endl;
return;
}
else {
cout << "库存不足!" << endl;
return;
}
}
}
cout << "未找到该书籍!" << endl;
}
// 归还书籍
void return_book(string name) {
for (auto& book : books) {
if (book.name == name) {
book.stock++;
cout << "归还成功!" << endl;
return;
}
}
cout << "未找到该书籍!" << endl;
}
// 显示库存
void show_stock() {
cout << "======库存清单======" << endl;
for (auto& book : books) {
cout << "书名:" << book.name << ",作者:" << book.author << ",出版社:" << book.publisher << ",出版年份:" << book.publish_year << ",库存:" << book.stock << endl;
}
cout << "====================" << endl;
}
private:
vector<Book> books; // 存储书籍信息
};
int main() {
Library library;
// 添加书籍
library.add_book("C++ Primer", "Lippman", "O'Reilly", 2013, 10);
library.add_book("Effective C++", "Scott Meyers", "Addison-Wesley", 2005, 5);
library.add_book("Thinking in Java", "Bruce Eckel", "Prentice Hall", 2006, 8);
// 显示库存
library.show_stock();
// 借书
library.borrow_book("C++ Primer");
library.borrow_book("C++ Primer");
library.borrow_book("C++ Primer");
// 显示库存
library.show_stock();
// 还书
library.return_book("C++ Primer");
// 显示库存
library.show_stock();
return 0;
}
```
在这个示例中,我们定义了一个`Book`结构体来存储书籍的信息,包括书名、作者、出版社、出版年份和库存。我们还定义了一个`Library`类,用于存储书籍信息,并提供添加书籍、借出书籍、归还书籍和显示库存等功能。在`main`函数中,我们创建了一个`Library`对象,添加了几本书,然后借了几本书和还了一本书,并显示库存情况。
当然,这只是一个简单的示例,实际的图书管理系统可能需要更多的功能和更复杂的逻辑,需要根据实际需求进行开发。
请你帮我写一份关于C++类库管理系统的需求分析
好的,以下是关于C++类库管理系统的需求分析:
1. 系统概述
C++类库管理系统是一款用于管理C++类库的软件,其主要功能包括:类库的添加、删除、查询、修改、分类、导出、导入等操作。通过这个系统,用户可以方便地管理自己的C++类库,提升代码重用性,提高开发效率。
2. 功能需求
2.1 类库管理
用户可以通过界面添加、删除、查询、修改、分类类库,并且可以对类库进行导入和导出操作。同时,用户可以对类库进行查看和编辑,以便更好地管理类库。
2.2 类管理
在类库中,用户可以添加、删除、查询、修改类,并且可以对类进行分类。同时,用户可以对类进行查看和编辑,以便更好地管理类。
2.3 函数管理
在类中,用户可以添加、删除、查询、修改函数,并且可以对函数进行分类。同时,用户可以对函数进行查看和编辑,以便更好地管理函数。
2.4 变量管理
在类中,用户可以添加、删除、查询、修改变量,并且可以对变量进行分类。同时,用户可以对变量进行查看和编辑,以便更好地管理变量。
2.5 代码生成
系统可以自动生成类库、类、函数和变量的代码,以便用户更方便地使用和调用。
3. 非功能需求
3.1 界面友好
系统的界面应该简洁明了,易于操作,用户可以轻松地完成各项任务。
3.2 系统安全
系统应该有一套完整的安全机制,保证用户信息和数据的安全性。
3.3 系统稳定性
系统应该具有较高的稳定性,避免出现意外崩溃等问题。
3.4 响应速度
系统应该具有较快的响应速度,避免用户等待时间过长。
以上是关于C++类库管理系统的需求分析,需求分析人员需要根据具体的项目情况进行细化和补充。
相关推荐
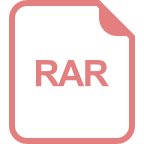
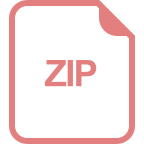








