C#把文件复制到指定路径下
时间: 2023-11-20 16:06:28 浏览: 33
你可以使用C#中的File类提供的Copy方法将文件复制到指定路径下。以下是示例代码:
```csharp
string sourceFilePath = @"C:\source\file.txt";
string destinationFilePath = @"C:\destination\file.txt";
File.Copy(sourceFilePath, destinationFilePath);
```
在上面的代码中,我们将源文件路径和目标文件路径存储在字符串变量sourceFilePath和destinationFilePath中,然后调用File.Copy方法将源文件复制到目标文件路径。请确保你有足够的权限对源文件和目标文件进行读写操作。
相关问题
C#把文件复制到指定文件夹
你可以使用C#中的File类和Directory类来实现文件复制和移动操作。以下是一个将文件复制到指定文件夹的示例:
```csharp
using System.IO;
// 源文件路径
string sourceFilePath = @"C:\example\source.txt";
// 目标文件夹路径
string targetFolderPath = @"C:\example\target";
// 复制文件到目标文件夹
File.Copy(sourceFilePath, Path.Combine(targetFolderPath, Path.GetFileName(sourceFilePath)));
```
在这个示例中,我们首先定义了源文件路径和目标文件夹路径。然后我们使用`File.Copy()`方法将源文件复制到目标文件夹中。`Path.Combine()`方法用于将目标文件夹路径与源文件名组合成完整的目标文件路径,`Path.GetFileName()`方法用于获取源文件的文件名。
你还可以使用`File.Move()`方法来移动文件到指定文件夹,方法与`File.Copy()`类似,但是它会删除源文件。如果你需要在移动文件时保留源文件,可以使用`File.Copy()`方法复制文件到目标文件夹,然后再使用`File.Delete()`方法删除源文件。
c#编程实现,将文件复制到指定路径,并修改复制后的文件名称
好的,下面是 C# 代码示例,可以实现将文件复制到指定路径,并修改复制后的文件名称:
``` csharp
using System.IO;
// 原文件路径和文件名
string srcFilePath = @"path\to\src\file";
string srcFileName = "src_file.txt";
// 目标路径和文件名
string dstFilePath = @"path\to\dst\directory";
string dstFileName = "dst_file.txt";
// 拼接出源文件的完整路径
string srcFullPath = Path.Combine(srcFilePath, srcFileName);
// 拼接出目标文件的完整路径
string dstFullPath = Path.Combine(dstFilePath, dstFileName);
// 复制文件到目标路径
File.Copy(srcFullPath, dstFullPath);
// 修改文件名称
File.Move(dstFullPath, Path.Combine(dstFilePath, "new_file_name.txt"));
```
在上面的示例中,我们使用了 `System.IO` 命名空间中的 `File` 类来复制和移动文件,并使用 `Path` 类的 `Combine` 方法来拼接文件路径。
相关推荐
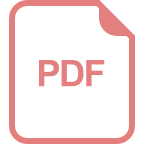












