python实现对word中每段话进行jieba分词存储 代码
时间: 2023-06-01 18:05:22 浏览: 183
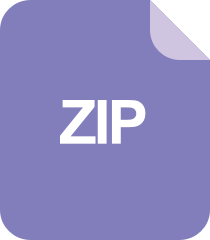
python使用jieba进行分词统计

可以使用Python的python-docx库读取Word文档,并使用jieba库进行中文分词,最后将分词结果存储到文件中。以下是示例代码:
```python
import docx
import jieba
doc = docx.Document('your_document.docx') # 替换为你的Word文档路径
with open('result.txt', 'w', encoding='utf-8') as f:
for para in doc.paragraphs:
seg_list = jieba.cut(para.text)
f.write(' '.join(seg_list))
f.write('\n')
```
该代码读取指定的Word文档,提取每个段落的文本,并使用jieba进行分词。分词结果以空格为分隔符保存在result.txt文件中。请注意,你需要先安装python-docx和jieba库。
阅读全文
相关推荐
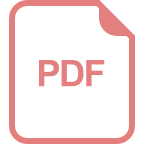
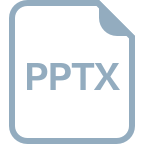














