c++ openssl读取RSA的公钥和私钥
时间: 2023-09-24 20:11:13 浏览: 59
以下是使用C++和OpenSSL库读取RSA公钥和私钥的示例代码:
```c++
#include <openssl/rsa.h>
#include <openssl/pem.h>
// 读取RSA公钥
RSA* read_public_key(const char* filename)
{
FILE* fp = fopen(filename, "r");
if (!fp) {
return NULL;
}
RSA* rsa = PEM_read_RSA_PUBKEY(fp, NULL, NULL, NULL);
fclose(fp);
return rsa;
}
// 读取RSA私钥
RSA* read_private_key(const char* filename, const char* password)
{
FILE* fp = fopen(filename, "r");
if (!fp) {
return NULL;
}
RSA* rsa = PEM_read_RSAPrivateKey(fp, NULL, NULL, (void*)password);
fclose(fp);
return rsa;
}
int main()
{
// 读取公钥
RSA* public_key = read_public_key("public_key.pem");
if (!public_key) {
// 处理读取公钥失败的情况
}
// 读取私钥
RSA* private_key = read_private_key("private_key.pem", "password");
if (!private_key) {
// 处理读取私钥失败的情况
}
// 使用公钥和私钥进行加解密等操作
// 释放公钥和私钥资源
RSA_free(public_key);
RSA_free(private_key);
return 0;
}
```
注意:如果私钥使用了密码保护,需要在调用`PEM_read_RSAPrivateKey`函数时传入密码。如果私钥没有密码保护,可以将最后一个参数设为NULL。
相关推荐
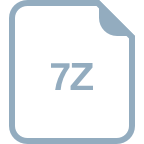
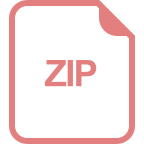














